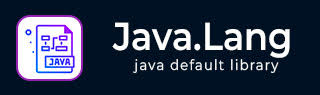
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String toUpperCase() 方法
描述
Java String toUpperCase() 方法用于将给定字符串的所有字符转换为大写字母。
此方法有两个多态变体,一个没有任何参数,另一个使用给定Locale 数据类型概述的标准将字符串转换为大写。这些方法的语法如下所示。
注意 - 请记住,大小写映射基于Character 类标准的 Unicode 标准版本。由于大小写映射不一定是 1:1 的,因此生成的新的字符串的长度可能与原始字符串的长度匹配,也可能不匹配。
语法
以下是Java String toUpperCase()方法的语法:
public String toUpperCase() or, public String toUpperCase(Locale locale)
参数
locale - 使用此区域设置的大小写转换规则。
返回值
此方法返回转换为大写的字符串。
将字符串转换为大写示例
以下示例演示了 Java String toUpperCase() 方法的使用,通过不传递任何参数将字符串的给定字符转换为大写字母:
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { // converts all lower case letters in to upper case letters String str1 = "This is TutorialsPoint"; System.out.println("string value = " + str1.toUpperCase()); str1 = "www.tutorialspoint.com"; System.out.println("string value = " + str1.toUpperCase()); } }
输出
如果编译并运行上面的程序,则输出将显示如下:
string value = THIS IS TUTORIALSPOINT string value = WWW.TUTORIALSPOINT.COM
使用 Locale 将字符串转换为大写示例
以下是一个使用 toUpperCase() 方法通过传递 Locale 参数将字符串中的字符转换为大写的示例:
package com.turialspoint; import java.util.Locale; public class StringDemo { public static void main(String[] args) { String str1 = "This is TutorialsPoint"; // using the default system Locale Locale defloc = Locale.getDefault(); // converts all lower case letters in to upper case letters System.out.println("string value = " + str1.toUpperCase(defloc)); str1 = "www.tutorialspoint.com"; System.out.println("string value = " + str1.toUpperCase(defloc)); } }
输出
如果编译并运行上述程序,它将产生以下结果:
string value = THIS IS TUTORIALSPOINT string value = WWW.TUTORIALSPOINT.COM
将包含非字母字符的字符串转换为大写示例
让我们创建一个另一个代码,该代码将生成包含字母、数字和符号的字符字符串。在这个程序中,我们将确定 toUpperCase() 方法是否会影响数字和符号等非字母字符:
package com.turialspoint; public class StringDemo { public static void main(String[] args) { String s = "Welcome to @!! Tutorials point 77!!"; System.out.println("The given string is: " + s); String toUpper = s.toUpperCase(); System.out.println("String after conversion is: " + toUpper); } }
输出
执行上述程序后,输出如下所示:
The given string is: Welcome to @!! Tutorials point 77!! String after conversion is: WELCOME TO @!! TUTORIALS POINT 77!!
使用 Locale 将字符串转换为大写示例
在下面的示例中,我们创建一个程序,通过将Locale对象作为参数传递给此方法,将字符串“Welcome to turialspoint”转换为大写字母:
package com.turialspoint; import java.util.Locale; public class StringDemo { public static void main(String[] args) { String s = new String("Welcome to turialspoint"); System.out.println("The given string is: " + s); // Create Locales with language "Eng" for English. Locale English = Locale.forLanguageTag("Eng"); System.out.println("Uppercase letters in english: " + s.toUpperCase(English)); } }
输出
上述代码的输出如下所示:
The given string is: Welcome to turialspoint Uppercase letters in turkish: WELCOME TO TURIALSPOINT Uppercase letters in english: WELCOME TO TURIALSPOINT
java_lang_string.htm
广告