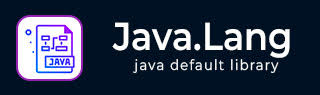
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 实用资源
- Java.lang - 讨论
Java - String trim() 方法
描述
Java String trim() 方法用于移除字符串开头和结尾的空格。它返回一个字符串副本,其中开头和结尾的空格已被省略;如果没有开头或结尾的空格,则此方法返回当前字符串。
此方法不会更改 String 对象的值。我们只需要将新的 String 对象赋值给一个新变量或重新赋值给原始 String,才能访问它。
注意 - 请记住,trim() 方法不会消除中间的空格。
语法
以下是Java String trim() 方法的语法:
public String trim()
参数
此方法不接受任何参数。
返回值
此方法返回此字符串的副本,其中已移除开头和结尾的空格;如果此字符串没有开头或结尾的空格,则返回此字符串本身。
从字符串中去除开头和结尾空格的示例
以下示例演示了如何使用 Java String trim() 方法从给定的字符串 " This is TutorialsPoint " 中移除开头和结尾的空格:
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { // string with leading and trailing white space String str = " This is TutorialsPoint "; System.out.print("Before trim = "); System.out.println(".." + str + ".."); // leading and trailing white space removed System.out.print("After trim = "); System.out.println(".." + str.trim() + ".."); } }
输出
如果您编译并运行上面的程序,则输出将显示如下:
Before trim = .. This is TutorialsPoint .. After trim = ..This is TutorialsPoint..
验证从字符串中去除开头和结尾空格后的长度示例
下面是另一个示例,它使用 Java 中的 trim() 方法移除所有开头和结尾的空格。在这里,我们验证了移除空格后的字符串长度:
package com.tutorialspoint; import java.util.Locale; public class StringDemo { public static void main(String[] args) { String str = " Tutorials Point "; System.out.println("The length of the string before trimming is: " + str.length()); System.out.println("The string without trimming is: " + str); String t = str.trim(); System.out.println("The length of the string after trimming is: " + t.length()); System.out.println("The string with trimming is:" + t); } }
输出
如果您编译并运行上面的程序,它将产生以下结果:
The length of the string before trimming is: 25 The string without trimming is: Tutorials Point The length of the string after trimming is: 15 The string with trimming is:Tutorials Point
检查从字符串中去除开头和结尾空格后的空格示例
让我们创建另一个代码,使用 if-else 条件来检查给定的字符串是否只包含空格:
package com.tutorialspoint; public class StringDemo { public static void main(String argvs[]) { String s = " Tutorials Point "; if ((s.trim()).length() > 0) { System.out.println("The string consists of characters other than the white spaces \n"); } else { System.out.println("The string consists of only white spaces \n"); } s = " "; if ((s.trim()).length() > 0) { System.out.println("The string consists of characters other than the white spaces \n"); } else { System.out.println("The string consists of only white spaces \n"); } } }
输出
运行上面的程序后,获得的输出如下:
The string consists of characters other than the white spaces The string consists of only white spaces
检查从字符串中去除开头和结尾空格后的哈希码示例
由于 Java 中的字符串是不可变的,因此使用 trim() 方法从字符串中移除空格时,会返回一个新字符串。如果 trim() 方法没有进行操作,则会返回对同一字符串的引用,如下例所示:
package com.tutorialspoint; public class StringDemo { public static void main(String argvs[]) { String s = " Tutorials Point "; String s1 = s.trim(); // the hashcode of s and s1 is different System.out.println("The hashcode of string s is: " + s.hashCode()); System.out.println("The hashcode of string s1 is: " + s1.hashCode()); String x = "calm"; String y = x.trim(); // the hashcode of x and y is the same System.out.println("The hashcode of string x is: " + x.hashCode()); System.out.println("The hashcode of string y is: " + y.hashCode()); } }
输出
上述代码的输出如下所示:
The hashcode of string s is: 932888837 The hashcode of string s1 is: -1622622651 The hashcode of string x is: 3045983 The hashcode of string y is: 3045983
java_lang_string.htm
广告