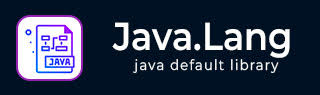
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StringBuffer codePointAt() 方法
Java 的StringBuffer codePointAt() 方法用于获取 StringBuffer 中指定索引处的字符(其 Unicode 代码点)。索引范围从 0 到 length() - 1。
注意 - 如果在给定索引处指定的字符值位于高代理范围,并且其后续索引小于此序列的长度,并且此后续索引处的字符值位于低代理范围,则返回对应于此代理对的补充代码点。否则,返回给定索引处的字符值。
语法
以下是 Java StringBuffer codePointAt() 方法的语法
public int codePointAt(int index)
参数
- index - 这是字符值的索引。
返回值
此方法返回索引处字符的代码点值。
示例:获取给定索引处的代码点
如果我们使用字母作为 CharSequence 初始化 StringBuffer 对象,则该方法返回 StringBuffer 参数索引处存在的字符。
以下示例显示了 Java StringBuffer codePointAt() 方法的使用。
package com.tutorialspoint; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("TUTORIALS"); System.out.println("buffer = " + buff); // returns the codepoint at index 5 int retval = buff.codePointAt(5); System.out.println("Character(unicode point) = " + retval); buff = new StringBuffer("amrood admin "); System.out.println("buffer = " + buff); // returns the codepoint at index 6 i.e whitespace character retval = buff.codePointAt(6); System.out.println("Character(unicode point) = " + retval); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
buffer = TUTORIALS Character(unicode point) = 73 buffer = amrood admin Character(unicode point) = 32
示例:获取给定索引处的代码点
在另一个示例中,我们创建一个 StringBuffer 对象并使用数字序列对其进行初始化。然后,使用索引作为其参数在此对象上调用该方法。
public class StringBufferCodePointAt { public static void main(String[] args) { StringBuffer obj = new StringBuffer("78343890"); int id = 5; System.out.println("The code point at index " + id + " is: " + obj.codePointAt(id)); } }
输出
一旦我们编译并运行上面的程序,输出将显示如下:
The code point at index 5 is: 56
示例:获取给定索引处的代码点
如果我们使用符号作为 CharSequence 初始化 StringBuffer 对象,并将索引作为参数传递,则该方法将返回该索引处存在的字符。
public class StringBufferCodePointAt { public static void main(String[] args) { StringBuffer obj = new StringBuffer("#$%^&*("); int id = 3; System.out.println("The code point at index " + id + " is: " + obj.codePointAt(id)); } }
输出
上述程序的输出打印为:
The code point at index 3 is: 94
示例:在获取负索引处的代码点时遇到异常
如果作为参数传递给此方法的索引无效(为负或大于字符序列长度),则会抛出异常。
public class StringBufferCodePointAt { public static void main(String[] args) { StringBuffer obj = new StringBuffer("Tutorialspoint"); int id = -2; System.out.println("The code point at index " + id + " is: " + obj.codePointAt(id)); //throws exception } }
异常
如果我们尝试编译并运行上述程序,则会抛出异常而不是打印输出:
Exception in thread "main" java.lang.StringIndexOutOfBoundsException: Index -2 out of bounds for length 14 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckIndex(Preconditions.java:106) at java.base/jdk.internal.util.Preconditions.checkIndex(Preconditions.java:302) at java.base/java.lang.String.checkIndex(String.java:4570) at java.base/java.lang.AbstractStringBuilder.codePointAt(AbstractStringBuilder.java:389) at java.base/java.lang.StringBuffer.codePointAt(StringBuffer.java:252) at StringBufferCodePointAt.main(StringBufferCodePointAt.java:11)
java_lang_stringbuffer.htm
广告