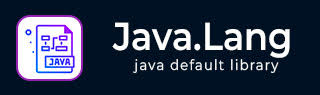
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StringBuffer codePointCount() 方法
Java 的StringBuffer codePointCount() 方法计算此序列指定文本范围内的 Unicode 代码点的数量。文本范围从指定的起始索引开始,扩展到倒数第二个索引处的字符。因此,文本范围的长度(以字符为单位)为结束索引 - 开始索引。
如果在给定文本范围内不存在 Unicode 代码点,则该方法不会抛出任何错误,只会打印“0”。
注意 - 未配对的代理代码点分别被视为一个单独的代码点。
语法
以下是 Java StringBuffer codePointCount() 方法的语法
public int codePointCount(int beginIndex, int endIndex)
参数
- beginIndex - 这是文本范围第一个字符的索引。
- endIndex - 这是文本范围最后一个字符之后的索引。
返回值
此方法返回指定文本范围内的 Unicode 代码点的数量。
示例:获取文本范围的长度
当我们将输入文本视为字母时,该方法会返回作为参数给出的文本范围的长度。
以下示例演示了 Java StringBuffer codePointCount() 方法的使用。
package com.tutorialspoint; public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("TUTORIALS"); System.out.println("buffer = " + buff); // returns the codepoint count from index 1 to 5 int retval = buff.codePointCount(1, 5); System.out.println("Count = " + retval); buff = new StringBuffer("7489042 "); System.out.println("buffer = " + buff); // returns the codepoint count from index 3 to 9 retval = buff.codePointCount(3, 9); System.out.println("Count = " + retval); buff = new StringBuffer("@#$%^&"); System.out.println("buffer = " + buff); // returns the codepoint count from index 2 to 4 retval = buff.codePointCount(2, 4); System.out.println("Count = " + retval); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
buffer = TUTORIALS Count = 4 buffer = 7489042 Count = 6 buffer = @#$%^& Count = 2
示例:获取没有有效代码点的文本范围的长度
当我们将输入文本视为没有有效代码点的字符时,该方法会返回零。
public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("/u1298139"); System.out.println("buffer = " + buff); // returns the codepoint count int retval = buff.codePointCount(0, 0); System.out.println("Count = " + retval); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
buffer = /u1298139 Count = 0
示例:在检查代码点数时遇到异常
但是,如果给定的索引参数超过或早于文本范围,则该方法会抛出 IndexOutOfBounds 异常。
public class StringBufferDemo { public static void main(String[] args) { StringBuffer buff = new StringBuffer("djk137"); System.out.println("buffer = " + buff); // returns the codepoint count from index 2 to 4 int retval = buff.codePointCount(-1, 9); System.out.println("Count = " + retval); } }
异常
如果我们编译并运行程序,则会抛出 IndexOutOfBounds 异常,而不是打印输出:
buffer = djk137 Exception in thread "main" java.lang.IndexOutOfBoundsException at java.lang.AbstractStringBuilder.codePointCount(AbstractStringBuilder.java:320) at java.lang.StringBuffer.codePointCount(StringBuffer.java:227)at StringBufferDemo.main(StringBufferDemo.java:11)
java_lang_stringbuffer.htm
广告