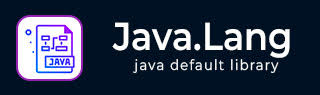
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StringBuffer delete() 方法
Java 的StringBuffer delete() 方法用于从序列中移除子字符串的元素。该方法从起始索引开始移除子字符串,直到最后一个索引之前的元素;也就是说,起始索引是包含的,而结束索引是不包含的。
但是,如果起始索引小于结束索引或大于创建的序列长度,则该方法会抛出 StringIndexOutOfBoundsException 异常。
注意 - 如果结束索引大于序列长度,则该方法会移除从起始索引到此序列末尾的所有字符。
语法
以下是 Java StringBuffer delete() 方法的语法。
public StringBuffer delete(int start, int end)
参数
- start - 这是起始索引,包含在内。
- end - 这是结束索引,不包含在内。
返回值
此方法返回此序列的子字符串。
示例:从 StringBuffer 中删除子字符串
如果 startIndex 值大于 0 且endIndex 值小于序列长度,则返回此序列的子字符串。
在下面的程序中,首先,我们使用值“Welcome to Tutorials Point”实例化StringBuffer 类。使用delete()方法,我们尝试删除给定范围内的字符串(startIndex = 5 和 endIndex = 10)。
public class StringBufferDemo { public static void main(String[] args) { //create a StringBuffer StringBuffer sb = new StringBuffer("Welcome to Tutorials Point"); System.out.println("Before deletion the string is: " + sb); //initialize the startInde and endIndex values int startIndex = 11; int endIndex = 21; System.out.println("The startIndex and endIndex values are: " + startIndex + " and " + endIndex); //using the delete() method StringBuffer new_str = sb.delete(startIndex, endIndex); System.out.println("After deletion the remaing string is: " + new_str); } }
输出
执行上述程序后,将产生以下结果:
Before deletion the string is: Welcome to Tutorials Point The startIndex and endIndex values are: 11 and 21 After deletion the remaing string is: Welcome to Point
示例:在从 StringBuffer 删除子字符串时遇到 StringIndexOutOfBoundsException
如果startIndex 值大于 endIndex 值,此方法将抛出StringIndexOutOfBoundsException。
在下面的示例中,我们使用值“JavaProgramming”创建一个StringBuffer对象。然后使用delete()方法,我们尝试删除给定范围内的字符串(其中 startIndex > endIndex)。
public class StringBufferDemo { public static void main(String[] args) { try { //create an object of the StringBuffer StringBuffer sb = new StringBuffer("JavaProgramming"); System.out.println("Before deletion the string is: " + sb); //initialize the startIndex and endIndex values int startIndex = 10;// greater than the endIndex value int endIndex = 5; System.out.println("The startIndex and endIndex values are: " + startIndex + " and " + endIndex); //using the delete() method System.out.println("After deletion rhe remaing string is: " + sb.delete(startIndex, endIndex)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
Before deletion the string is: JavaProgramming The startIndex and endIndex values are: 10 and 5 java.lang.StringIndexOutOfBoundsException: Range [10, 5) out of bounds for length 15 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckFromToIndex(Preconditions.java:112) at java.base/jdk.internal.util.Preconditions.checkFromToIndex(Preconditions.java:349) at java.base/java.lang.AbstractStringBuilder.delete(AbstractStringBuilder.java:904) at java.base/java.lang.StringBuffer.delete(StringBuffer.java:475) at StringBufferDemo.main(StringBufferDemo.java:12) Exception: java.lang.StringIndexOutOfBoundsException: Range [10, 5) out of bounds for length 15
示例:在从 StringBuffer 删除子字符串时遇到 IndexOutOfBoundsException
如果 startIndex 包含负值,则 delete() 方法将抛出IndexOutOfBoundsException。
在此示例中,我们使用值“TutorialsPoint”创建一个StringBuffer。然后,我们尝试使用delete()方法删除给定范围内的字符串(其中 startIndex 为负值-2)。
public class StringBufferDemo { public static void main(String[] args) { try { //create an object of the StringBuffer StringBuffer sb = new StringBuffer("TutorialsPoint"); System.out.println("Before deletion the string is: " + sb); //initialize the startIndex and endIndex values int startIndex = -2;// holds negative value int endIndex = 5; System.out.println("The startIndex and endIndex values are: " + startIndex + " and " + endIndex); //using the delete() method System.out.println("After deletion rhe remaing string is: " + sb.delete(startIndex, endIndex)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
上述程序产生以下结果:
Before deletion the string is: TutorialsPoint The startIndex and endIndex values are: -2 and 5 java.lang.StringIndexOutOfBoundsException: Range [-2, 5) out of bounds for length 14 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckFromToIndex(Preconditions.java:112) at java.base/jdk.internal.util.Preconditions.checkFromToIndex(Preconditions.java:349) at java.base/java.lang.AbstractStringBuilder.delete(AbstractStringBuilder.java:904) at java.base/java.lang.StringBuffer.delete(StringBuffer.java:475) at StringBufferDemo.main(StringBufferDemo.java:14) Exception: java.lang.StringIndexOutOfBoundsException: Range [-2, 5) out of bounds for length 14