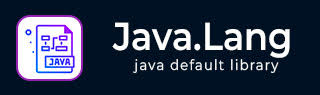
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StringBuffer deleteCharAt() 方法
java StringBuffer deleteCharAt() 方法用于移除此序列中指定位置处的字符。此序列将缩短一个字符。
在 Java 中,索引指的是当前序列的字符位置。基本上,charAt() 方法用于检索指定索引处的字符。deleteCharAt() 方法接受一个整数参数,该参数保存字符索引的值。如果索引值为负数或大于给定序列长度,则会抛出异常。
语法
以下是 Java StringBuffer deleteCharAt() 方法的语法:
public StringBuffer deleteCharAt(int index)
参数
index - 要移除的字符的索引。
返回值
此方法返回给定序列的子字符串。
示例:从 StringBuffer 中删除子字符串
如果索引值非负且小于序列长度,则此方法返回给定序列的新子字符串。
在以下示例中,我们使用值“Java lang package”创建 StringBuffer 类的一个对象。使用 delete() 方法,我们尝试删除指定索引 3处的字符。
public class StringBufferDemo { public static void main(String[] args) { //create an object of the StringBuffer StringBuffer sb = new StringBuffer("Java lang package"); System.out.println("Before deletion the string is: " + sb); //initialize the index value int index = 3; System.out.println("The given index value is: " + index); //using the deleteCharAt() method System.out.println("After deletion the character at the specified index " + index + " index is: " + sb.deleteCharAt(index)); } }
输出
执行上述程序后,将产生以下结果:
Before deletion the string is: Java lang package The given index value is: 3 After deletion the character at the specified index 3 index is: Jav lang package
示例:在从 StringBuffer 中删除子字符串时遇到 StringIndexOutOfBoundsException
如果索引值大于给定序列长度,则此方法将抛出StringIndexOutOfBoundsException。
在此程序中,我们使用值“JavaProgramming”实例化StringBuffer 类。使用deleteCharAt() 方法,我们尝试删除指定索引 30处的字符,该值大于给定序列。
public class Delete { public static void main(String[] args) { try { //create an object of the StringBuffer StringBuffer sb = new StringBuffer("JavaProgramming"); System.out.println("Before deletion the string is: " + sb); //initialize the index value int index = 30;// value is greater than the sequence length System.out.println("The given index value is: " + index); //using the deleteCharAt() method System.out.println("After deletion rhe remaing string is: " + sb.deleteCharAt(index)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
Before deletion the string is: JavaProgramming The given index value is: 30 java.lang.StringIndexOutOfBoundsException: Index 30 out of bounds for length 15 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckIndex(Preconditions.java:106) at java.base/jdk.internal.util.Preconditions.checkIndex(Preconditions.java:302) at java.base/java.lang.String.checkIndex(String.java:4557) at java.base/java.lang.AbstractStringBuilder.deleteCharAt(AbstractStringBuilder.java:957) at java.base/java.lang.StringBuffer.deleteCharAt(StringBuffer.java:486) at Delete.main(Delete.java:13) Exception: java.lang.StringIndexOutOfBoundsException: Index 30 out of bounds for length 15
示例:在从 StringBuffer 中删除子字符串时遇到 StringIndexOutOfBoundsException
如果索引值包含负值,则在调用此方法时,会抛出StringIndexOutOfBoundsException。
在以下示例中,我们创建一个值为“TutorialsPoint”的StringBuffer。使用deleterCharAt() 方法,我们尝试删除指定索引 -1处的字符。
public class Demo { public static void main(String[] args) { try { //create an object of the StringBuffer StringBuffer sb = new StringBuffer("TutorialsPoint"); System.out.println("Before deletion the string is: " + sb); //initialize the index value int index = -1;// holds the negative value System.out.println("The given index value is: " + index); //using the deleteCharAt() method System.out.println("After deletion rhe remaing string is: " + sb.deleteCharAt(index)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
上述程序产生以下结果:
Before deletion the string is: TutorialsPoint The given index value is: -1 java.lang.StringIndexOutOfBoundsException: Index -1 out of bounds for length 14 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckIndex(Preconditions.java:106) at java.base/jdk.internal.util.Preconditions.checkIndex(Preconditions.java:302) at java.base/java.lang.String.checkIndex(String.java:4557) at java.base/java.lang.AbstractStringBuilder.deleteCharAt(AbstractStringBuilder.java:957) at java.base/java.lang.StringBuffer.deleteCharAt(StringBuffer.java:486) at Demo.main(Demo.java:13) Exception: java.lang.StringIndexOutOfBoundsException: Index -1 out of bounds for length 14
java_lang_stringbuffer.htm
广告