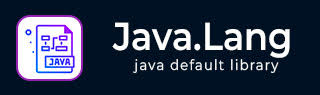
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StringBuffer getChars() 方法
Java StringBuffer getChars() 方法将此序列中的字符复制到目标字符数组dst中。在 Java 中,数组是一个包含相同数据类型元素的对象。
要复制的第一个字符位于索引srcBegin处;要复制的最后一个字符位于索引srcEnd - 1处。要复制的字符总数为srcEnd - srcBegin。这些字符被复制到从索引 dstBegin 开始并以索引dstbegin + (srcEnd-srcBegin) – 1结束的 dst 的子数组中。
语法
以下是Java StringBuffer getChars() 方法的语法:
public void getChars(int srcBegin, int srcEnd, char[] dst, int dstBegin)
参数
- srcBegin − 表示从此偏移量开始复制。
- srcEnd − 表示在此偏移量停止复制。
- dst − 这是要将数据复制到的数组。
- dstBegin − 这是 dst 中的偏移量。
返回值
此方法不返回值。
示例:获取子字符串的字符数组
如果目标字符数组不为空,并且索引值是正数,则getChars() 方法会将此序列的字符复制到字符数组中。
在下面的程序中,我们使用值“Welcome to TutorialsPoint”创建了一个StringBuffer 类的对象。使用getChars() 方法,我们尝试将字符的值复制到目标数组中,在srcBegin 索引 3、srcEnd 索引 5和dstBegin 索引 0处。
public class Demo { public static void main(String[] args) { // creating an object of the StringBuffer class StringBuffer sb = new StringBuffer("Welcome to TutorialsPoint"); System.out.println("The given string value is: " + sb); //create an array of character char dst[] = {'A','B','C'}; System.out.print("The characrer array elements are: "); for(int i = 0; i<dst.length; i++) { System.out.print(dst[i] + " "); } //initialize the srcBegin, srcEnd, dstBegin int srcBegin = 3; int srcEnd = 5; int dstBegin = 0; System.out.println("\nThe values of srcBegin, srcEnd, and dstBegin are: " + srcBegin + " , " + srcEnd + " and " + dstBegin); //using the getChars() method sb.getChars(srcBegin, srcEnd, dst, dstBegin); System.out.print("The new copied character array elements are: "); for(int i = 0; i<dst.length; i++) { System.out.print(dst[i] + " "); } } }
输出
执行上述程序后,将产生以下结果:
The given string value is: Welcome to TutorialsPoint The characrer array elements are: A B C The values of srcBegin, srcEnd, and dstBegin are: 3 , 5 and 0 The new copied character array elements are: c o C
示例:获取空子字符串的字符数组时遇到 NullPointerException
如果目标字符数组为null,则 getChars() 方法会抛出NullPointerException。
在下面的程序中,我们使用值“Java Programming”实例化了StringBuffer 类。然后,我们创建了一个值为 null 的字符数组。使用getChars() 方法,我们尝试将此序列的字符复制到包含 null 值的字符数组中。
public class Demo { public static void main(String[] args) { try { // instantiating the StringBuffer StringBuffer sb = new StringBuffer("Java Programming"); System.out.println("The given string value is: " + sb); //create an array of character char dst[] = null; System.out.println("The characrer array elements are: " + dst); //initialize the srcBegin, srcEnd, dstBegin int srcBegin = 0; int srcEnd = 5; int dstBegin = 0; System.out.println("The values of srcBegin, srcEnd, and dstBegin are: " + srcBegin + " , " + srcEnd + " and " + dstBegin); //using the getChars() method sb.getChars(srcBegin, srcEnd, dst, dstBegin); System.out.println("The new copied character array elements are: " + dst); } catch(NullPointerException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
The given string value is: Java Programming The characrer array elements are: null The values of srcBegin, srcEnd, and dstBegin are: 0 , 5 and 0 java.lang.NullPointerException: Cannot read the array length because "dst" is null at java.base/java.lang.AbstractStringBuilder.getChars(AbstractStringBuilder.java:499) at java.base/java.lang.StringBuffer.getChars(StringBuffer.java:289) at Demo.main(Demo.java:21) Exception: java.lang.NullPointerException: Cannot read the array length because "dst" is null
示例:获取无效索引的字符数组时遇到 IndexOutOfBoundsException
如果给定的 srcBegin 和 dstBegin 值为负数,则此方法会抛出IndexOutOfBoundsException。
在下面的示例中,我们使用值“HelloWorld”创建了一个StringBuffer 类的对象。使用getChars() 方法,我们尝试将此序列的字符复制到字符数组中,在 srcBegin 索引和 dstBegin 索引-1处。
public class Demo { public static void main(String[] args) { try { // instantiating the StringBuffer StringBuffer sb = new StringBuffer("HelloWorld"); System.out.println("The given string value is: " + sb); //create an array of character char dst[] = {'a','b','c'}; System.out.println("The characrer array elements are: " + dst); //initialize the srcBegin, srcEnd, dstBegin int srcBegin = -1; int srcEnd = 5; int dstBegin = -1; System.out.println("The values of srcBegin, srcEnd, and dstBegin are: " + srcBegin + " , " + srcEnd + " and " + dstBegin); //using the getChars() method sb.getChars(srcBegin, srcEnd, dst, dstBegin); System.out.println("The new copied character array elements are: " + dst); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
上述程序产生以下结果:
The given string value is: HelloWorld The characrer array elements are: [C@76ed5528 The values of srcBegin, srcEnd, and dstBegin are: -1 , 5 and -1 java.lang.StringIndexOutOfBoundsException: Range [-1, 5) out of bounds for length 10 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckFromToIndex(Preconditions.java:112) at java.base/jdk.internal.util.Preconditions.checkFromToIndex(Preconditions.java:349) at java.base/java.lang.AbstractStringBuilder.getChars(AbstractStringBuilder.java:497) at java.base/java.lang.StringBuffer.getChars(StringBuffer.java:289) at Demo.main(Demo.java:21) Exception: java.lang.StringIndexOutOfBoundsException: Range [-1, 5) out of bounds for length 10