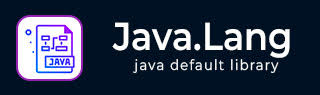
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StringBuffer indexOf() 方法
Java StringBuffer indexOf() 方法用于检索指定字符在字符串或 StringBuffer 中第一次出现的索引,该方法区分大小写,这意味着字符串“A”和“a”是两个不同的值。
indexOf() 方法接受一个字符串参数,该参数保存子字符串的值。它返回一个整数值,如果当前字符串中不存在给定值,则返回-1。如果给定的字符串值为null,则该方法会抛出异常。
indexOf() 方法有两个多态变体,它们具有不同的参数,例如 - 字符串和 fromIndex(下面是这两个多态变体的语法)。
语法
以下是Java StringBuffer indexOf() 方法的语法:
public int indexOf(String str) public int indexOf(String str, int fromIndex)
参数
- str − 这是子字符串值。
- fromIndex − 这是开始搜索的索引。
返回值
如果字符串参数作为子字符串出现在此对象中,则此方法返回第一个此类子字符串的第一个字符的索引。如果它没有作为子字符串出现,则返回 -1。
如果将值传递给fromIndex参数,则此方法将返回指定子字符串的第一次出现处的索引,从指定的索引开始。
示例:获取子字符串的索引
如果给定的字符串值不为 null并且存在于当前字符串中,则此方法返回其位置。
在这个程序中,我们正在创建一个值为“Welcome to TutorialsPoint”的StringBuffer对象。然后,使用indexOf()方法,我们尝试检索子字符串“to”的索引。
package com.tutorialspoint.StringBuffer; public class Index { public static void main(String[] args) { //instantiating the StringBuffer StringBuffer sb = new StringBuffer("Welcome to TutorialsPoint"); System.out.println("The given string is: " + sb); //initialize the substring String str = "to"; System.out.println("The sustring is: " + str); //using the indexOf() method System.out.println("The position of " + str + " is: " + sb.indexOf(str)); } }
输出
执行上述程序后,将产生以下结果:
The given string is: Welcome to TutorialsPoint The sustring is: to The position of to is: 8
示例:获取 null 的索引时遇到 NullPointerException
如果给定的字符串值为null,则indexOf()方法将抛出NullPointerException。
在下面的示例中,我们使用 null 值实例化一个StringBuffer。使用indexOf()方法,我们尝试检索StringBuffer中指定字符第一次出现的位置。由于给定的字符串值为null,因此该方法会抛出异常。
package com.tutorialspoint.StringBuffer; public class Index { public static void main(String[] args) { try { //instantiating the StringBuffer StringBuffer sb = new StringBuffer(null); System.out.println("The given string is: " + sb); //initialize the substring String str = "hello"; System.out.println("The sustring is: " + str); //using the indexOf() method System.out.println("The position of " + str + " is: " + sb.indexOf(str)); } catch(NullPointerException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
java.lang.NullPointerException: Cannot invoke "String.length()" because "str" is null at java.base/java.lang.AbstractStringBuilder.(AbstractStringBuilder.java:105) at java.base/java.lang.StringBuffer. (StringBuffer.java:131) at com.tutorialspoint.StringBuffer.Index.main(Index.java:6) Exception: java.lang.NullPointerException: Cannot invoke "String.length()" because "str" is null
示例:获取不存在的子字符串的索引
如果在 StringBuffer 中找不到给定的字符串值,则 indexOf() 方法返回-1。
在下面的程序中,我们使用值为“TutorialsPoint”的StringBuffer创建一个对象。然后,使用indexOf()方法,我们尝试检索给定StringBuffer中“tutorix”的位置。
package com.tutorialspoint.StringBuffer; public class Index { public static void main(String[] args) { //instantiating the StringBuffer StringBuffer sb = new StringBuffer("TutorialsPoint"); System.out.println("The given string is: " + sb); //initialize the substring String str = "tutorix"; System.out.println("The sustring is: " + str); //using the indexOf() method System.out.println("The position of " + str + " is: " + sb.indexOf(str)); } }
输出
上述程序产生以下输出:
The given string is: TutorialsPoint The sustring is: tutorix The position of tutorix is: -1
示例:获取子字符串的索引
如果给定的字符串值不为 null并且 fromIndex 值为正数,则 indexOf() 方法返回子字符串的位置。
在这个例子中,我们使用值为“Java Programming Language”的StringBuffer进行实例化。然后使用indexOf()方法,我们尝试从索引10检索“language”子字符串的位置。
package com.tutorialspoint.StringBuffer; public class Index { public static void main(String[] args) { //instantiating the StringBuffer StringBuffer sb = new StringBuffer("Java Programming Language"); System.out.println("The given string is: " + sb); //initialize the substring and fromIndex value String str = "Language"; int fromIndex = 10; System.out.println("The sustring is: " + str); System.out.println("The fromIndex value is: " + fromIndex); //using the indexOf() method System.out.println("The position of " + str + " is: " + sb.indexOf(str, fromIndex)); } }
输出
执行上述程序后,程序将产生以下输出:
The given string is: Java Programming Language The sustring is: Language The fromIndex value is: 10 The position of Language is: 17