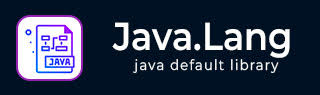
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 实用资源
- Java.lang - 讨论
Java StringBuffer lastIndexOf() 方法
Java StringBuffer lastIndexOf() 方法用于在 StringBuffer 对象中检索给定字符串最右侧出现的索引。索引指的是字符在字符串中的位置。
lastIndexOf() 方法接受一个字符串参数,该参数包含子字符串的值。如果给定的字符串为 null,则会抛出异常。该方法返回一个整数值,如果未找到子字符串,则返回 -1。
lastIndexOf() 方法有两个多态变体,具有不同的参数,例如 - String 和 fromIndex(以下是两种多态变体的语法)。
语法
以下是 Java StringBuffer lastIndexOf() 方法的语法:
public int lastIndexOf(String str) public int lastIndexOf(String str, int fromIndex)
参数
- str − 这是要搜索的子字符串。
- fromIndex − 这是开始搜索的索引。
返回值
如果字符串参数在一个或多个次数作为子字符串出现在此对象中,则返回最后一个此类子字符串的第一个字符的索引。如果它没有作为子字符串出现,则返回 -1。
如果将fromIndex值作为参数与字符串一起传递,则此方法返回在此序列中指定子字符串最后一次出现的索引。
示例:获取子字符串的最后一个索引
如果给定的字符串值不为 null,则 lastIndexOf() 方法返回子字符串的索引值。
在下面的程序中,我们用值“Welcome to TutorialsPoint”实例化StringBuffer。使用lastIndexOf()方法,我们试图检索“to”子字符串的索引。
public class Index { public static void main(String[] args) { // instantiating the StringBuffer StringBuffer sb = new StringBuffer("Welcome to TutorialsPoint"); System.out.println("The given string is: " + sb); // initialize the substring and fromIndex value String str = "to"; System.out.println("The substring is: " + str); // using the indexOf() method System.out.println("The index value of '" + str + "' is: " + sb.lastIndexOf(str)); } }
输出
执行上述程序后,将产生以下结果:
The given string is: Welcome to TutorialsPoint The substring is: to The index value of 'to' is: 13
示例:获取 null 子字符串的最后一个索引时遇到 NullPointerException
如果给定的字符串值为null,则 lastIndexOf() 方法将抛出NullPointerException。
在下面的程序中,我们使用值“HelloWorld”创建一个StringBuffer对象。使用lastIndexOf()方法,我们试图检索 null 值的索引。
public class Index { public static void main(String[] args) { try { //instantiating the StringBuffer StringBuffer sb = new StringBuffer("HelloWorld"); System.out.println("The given string is: " + sb); //initialize the substring and fromIndex value String str = null; int fromIndex = 5; System.out.println("The sustring is: " + str); System.out.println("The fromIndex value is: " + fromIndex); //using the indexOf() method System.out.println("The position of " + str + " is: " + sb.lastIndexOf(str, fromIndex)); } catch(NullPointerException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
The given string is: HelloWorld The sustring is: null The fromIndex value is: 5 java.lang.NullPointerException: Cannot read field "value" because "tgtStr" is null at java.base/java.lang.String.lastIndexOf(String.java:2628) at java.base/java.lang.AbstractStringBuilder.lastIndexOf(AbstractStringBuilder.java:1521) at java.base/java.lang.StringBuffer.lastIndexOf(StringBuffer.java:698) at Index.main(Index.java:15) Exception: java.lang.NullPointerException: Cannot read field "value" because "tgtStr" is null
示例:获取不存在的子字符串的最后一个索引
如果给定的字符串值不为 null,但fromIndex 值为负数,则此方法返回-1。
在下面的示例中,我们用值“Java Programming Language”实例化StringBuffer。然后,使用lastIndexOf()方法,我们试图从索引-1检索“Programming”子字符串的索引。
public class Index { public static void main(String[] args) { //instantiating the StringBuffer StringBuffer sb = new StringBuffer("Java Programming Language"); System.out.println("The given string is: " + sb); //initialize the substring and fromIndex value String str = "Programming"; int fromIndex = -1; System.out.println("The sustring is: " + str); System.out.println("The fromIndex value is: " + fromIndex); //using the indexOf() method System.out.println("The position of " + str + " is: " + sb.lastIndexOf(str, fromIndex)); } }
输出
上述程序产生以下结果:
The given string is: Java Programming Language The sustring is: Programming The fromIndex value is: -1 The position of Programming is: -1
示例:获取子字符串的最后一个索引
如果 fromIndex 值为正数,并且大于子字符串索引值,则 lastIndexOf() 方法返回子字符串的索引值。
在下面的程序中,我们创建一个值为“TutorialsPoint”的StringBuffer。使用lastIndexof()方法,我们试图从索引10检索“als”子字符串的索引。
public class Index { public static void main(String[] args) { //instantiating the StringBuffer StringBuffer sb = new StringBuffer("TutorialsPoint"); System.out.println("The given string is: " + sb); //initialize the substring and fromIndex value String str = "als"; int fromIndex = 10; System.out.println("The sustring is: " + str); System.out.println("The fromIndex value is: " + fromIndex); //using the indexOf() method System.out.println("The position of " + str + " is: " + sb.lastIndexOf(str, fromIndex)); } }
输出
执行上述程序后,将产生以下输出:
The given string is: TutorialsPoint The sustring is: als The fromIndex value is: 10 The position of als is: 6