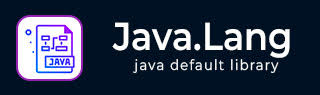
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StringBuffer length() 方法
Java StringBuffer length() 方法用于获取 StringBuffer 对象的长度。长度简单来说就是字符串/StringBuffer 中字符的数量。如果当前 StringBuffer 对象为空,则此方法返回零。
length() 方法不接受任何参数。在检索字符串长度时,它不会抛出任何异常。
注意 - 如果字符串为空字符串但包含一些空格,则 length() 方法会计算空格并将它们作为字符串的长度返回。
语法
以下是 Java StringBuffer length() 方法的语法:
public int length()
参数
它不接受任何参数。
返回值
此方法返回当前由该对象表示的字符序列的长度。
示例:获取 StringBuffer 字符串的长度
如果 StingBuilder 对象的值不为 null,则此方法返回字符串的长度。
在以下示例中,我们使用值“TutorialsPoint”创建了一个StringBuffer对象。使用length()方法,我们检索当前 StringBuffer 对象的长度。
public class Demo { public static void main(String[] args) { //creating an object of the StringBuffer StringBuffer sb = new StringBuffer("TutorialsPoint"); System.out.println("The given string is: " + sb); //using the length() method int length = sb.length(); System.out.println("The length of the '" + sb + "' is: " + length); } }
输出
执行上述程序后,将产生以下结果:
The given string is: TutorialsPoint The length of the 'TutorialsPoint' is: 14
示例:获取 StringBuffer 空字符串的长度
如果当前 StringBuffer 为空字符串,则 length() 方法返回0。
在以下程序中,我们使用空值实例化StringBuffer。使用length()方法,我们尝试检索此 StringBuffer 对象的长度。
import java.util.stream.IntStream; public class Demo { public static void main(String[] args) { //creating an object of the StringBuffer StringBuffer sb = new StringBuffer(""); System.out.println("The given string is: " + sb); //using the length() method int length = sb.length(); System.out.println("The length of the '" + sb + "' is: " + length); } }
输出
以下是上述程序的输出:
The given string is: The length of the '' is: 0
示例:获取 StringBuffer 字符串的长度
在以下示例中,我们使用值“HelloWorld”创建了一个StringBuffer对象。然后,我们使用 for 循环遍历字符串的length()。使用charAt()方法,我们尝试打印当前对象的每个字符。
public class Demo { public static void main(String[] args) { //creating an object of the StringBuffer StringBuffer sb = new StringBuffer("HelloWorld"); System.out.println("The given string is: " + sb); //using the length() method int length = sb.length(); System.out.println("The length of the '" + sb + "' is: " + length); //using for loop System.out.print("The string characters are: "); for(int i = 0; i<length; i++) { //using the charAt() method System.out.print(sb.charAt(i) + " "); } } }
输出
上述程序产生以下结果:
The given string is: HelloWorld The length of the 'HelloWorld' is: 10 The string characters are: H e l l o W o r l d
示例:获取 StringBuffer 包含空格的字符串的长度
如果当前对象只包含一些空格,则 length() 方法会计算空格并将其作为字符串长度返回。
在以下程序中,我们使用空值实例化StringBuffer,但它包含4 个空格。使用length()方法,我们尝试检索其长度。
public class Demo { public static void main(String[] args) { //instantiating StringBuffer StringBuffer sb = new StringBuffer(" "); System.out.println("The given string is: " + sb); //using the length() method int length = sb.length(); System.out.println("The length of the '" + sb + "' is: " + length); } }
输出
执行上述程序后,将产生以下输出:
The given string is: The length of the ' ' is: 3