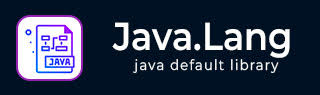
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包扩展
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StringBuffer replace() 方法
Java StringBuffer replace() 方法用于将 StringBuffer 对象的子字符串中的字符替换为指定字符串中的字符。子字符串从指定的开始位置开始,扩展到索引 end - 1处的字符,如果不存在这样的字符,则扩展到序列的末尾。
replace() 方法接受三个参数作为整数和字符串,分别保存start、end 和 str的值。如果开始索引值为负或大于字符串长度,则会抛出异常。
语法
以下是Java StringBuffer replace() 方法的语法:
public StringBuffer replace(int start, int end, String str)
参数
start - 这是开始索引,包含在内。
end - 这是结束索引,不包含在内。
str - 这是将替换先前内容的字符串。
返回值
此方法返回此对象。
示例:用另一个字符串替换子字符串
如果给定的开始索引值为正,并且小于字符串长度,则 replace() 方法将替换指定字符串中的字符。
在下面的程序中,我们使用值“Java Programming”实例化StringBuffer 类。然后,使用replace() 方法,我们尝试替换此序列中子字符串“Language”的字符,该子字符串的startIndex 为 5,endIndex 为 16。
public class Replace { public static void main(String[] args) { //instantiate the StringBuffer class StringBuffer sb = new StringBuffer("Java Programming"); System.out.println("The given String is: " + sb); //initialize the startIndex, endIndex, and string value int startIndex = 5; int endIndex = 16; String str = "Language"; System.out.println("The given startIndex and endIndex values are: " + startIndex + " and " + endIndex); System.out.println("The given sub-string is: " + str); //using the replace() method System.out.println("After replace the string: " + sb.replace(startIndex, endIndex, str)); } }
输出
执行上述程序后,将产生以下结果:
The given String is: Java Programming The given startIndex and endIndex values are: 5 and 16 The given sub-string is: Language After replace the string: Java Language
示例:在用另一个字符串替换子字符串时遇到 IndexOutOfBoundException
如果给定的startIndex 值为负,则 replace() 方法将抛出IndexOutOfBoundException。
在下面的示例中,我们使用值“TutorialsPoint”创建一个StringBuffer 类的对象。使用replace() 方法,我们尝试替换此序列中子字符串“Point”的字符,该子字符串的startIndex 为 -1,endIndex 为 10。
public class Replace { public static void main(String[] args) { try { //create an object StringBuffer class StringBuffer sb = new StringBuffer("TutorialsPoint"); System.out.println("The given String is: " + sb); //initialize the startIndex, endIndex, and string value int startIndex = -1; int endIndex = 10; String str = "India"; System.out.println("The given startIndex and endIndex values are: " + startIndex + " and " + endIndex); System.out.println("The given sub-string is: " + str); //using the replace() method System.out.println("After replace the string: " + sb.replace(startIndex, endIndex, str)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
The given String is: TutorialsPoint The given startIndex and endIndex values are: -1 and 10 The given sub-string is: India java.lang.StringIndexOutOfBoundsException: Range [-1, 10) out of bounds for length 14 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckFromToIndex(Preconditions.java:112) at java.base/jdk.internal.util.Preconditions.checkFromToIndex(Preconditions.java:349) at java.base/java.lang.AbstractStringBuilder.replace(AbstractStringBuilder.java:987) at java.base/java.lang.StringBuffer.replace(StringBuffer.java:497) at Replace.main(Replace.java:16) Exception: java.lang.StringIndexOutOfBoundsException: Range [-1, 10) out of bounds for length 14
示例:在用另一个字符串替换子字符串时遇到 IndexOutOfBoundException
如果给定的开始索引值大于字符串长度,则此方法将抛出IndexOutOfBoundException。
在此示例中,我们使用值“hello”实例化StringBuffer 类。使用replace() 方法,我们尝试替换此序列中子字符串“llo”的字符,该子字符串的startIndex 为 10,endIndex 为 5。
public class Replace { public static void main(String[] args) { try { //instantiate the StringBuffer class StringBuffer sb = new StringBuffer("hello"); System.out.println("The given String is: " + sb); //initialize the startIndex, endIndex, and string value int startIndex = 10; int endIndex = 5; String str = "world"; System.out.println("The given startIndex and endIndex values are: " + startIndex + " and " + endIndex); System.out.println("The given sub-string is: " + str); //using the replace() method System.out.println("After replace the string: " + sb.replace(startIndex, endIndex, str)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
上述程序产生以下输出:
The given String is: hello The given startIndex and endIndex values are: 10 and 5 The given sub-string is: world java.lang.StringIndexOutOfBoundsException: Range [10, 5) out of bounds for length 5 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckFromToIndex(Preconditions.java:112) at java.base/jdk.internal.util.Preconditions.checkFromToIndex(Preconditions.java:349) at java.base/java.lang.AbstractStringBuilder.replace(AbstractStringBuilder.java:987) at java.base/java.lang.StringBuffer.replace(StringBuffer.java:497) at Replace.main(Replace.java:16) Exception: java.lang.StringIndexOutOfBoundsException: Range [10, 5) out of bounds for length 5