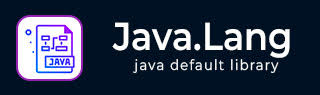
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StringBuffer setCharAt() 方法
**Java StringBuffer setCharAt()** 方法用于在 StringBuffer 对象的指定索引处添加/插入字符。**索引**指的是给定序列中字符的位置。我们作为索引参数传递的值必须大于或等于 0,且小于此序列的长度。
**setCharAt()** 方法接受两个参数作为整数和字符,分别保存**索引**和**ch**的值。如果索引值为负数,则会抛出异常。
语法
以下是 **Java StringBuffer setCharAt()** 方法的语法:-
public void setCharAt(int index, char ch)
参数
- **index** - 这是 char 值的索引。
- **ch** - 这是新的字符。
返回值
此方法不返回值。
示例:在给定索引处设置字符串中的字符
如果给定的索引值为**正数**,并且**小于字符串长度**,则 setCharAt() 方法会在指定索引处设置字符。
在以下程序中,我们使用值**“Tutorix”**实例化**StringBuffer 类**。使用**setCharAt()** 方法,我们尝试在指定索引**0**处设置字符**‘P’**。
public class SetChar { public static void main(String[] args) { //instantiate the StringBuffer class StringBuffer sb = new StringBuffer("Tutorix"); System.out.println("The given string is: " + sb); //initialize the index and ch values int index = 0; char ch = 'P'; System.out.println("The initialize index and ch values are: " + index + " and " + ch); //using the setCharAt() method sb.setCharAt(index, ch); System.out.println("After setting the character the string is: " + sb); } }
输出
执行上述程序后,将产生以下结果:-
The given string is: Tutorix The initialize index and ch values are: 0 and P After setting the character the string is: Putorix
示例:在给定索引处设置字符串中的字符时遇到 IndexOutOfBoundsException
如果给定的索引值为**负数**,则 setCharAt() 方法会抛出**IndexOutOfBoundsException**。
在以下程序中,我们使用值**“Hello”**创建**StringBuffer 类**的对象。使用**setCharAt()** 方法,我们尝试在指定索引**-1**处设置字符**‘o’**。
public class SetChar { public static void main(String[] args) { try { //create an object of the StringBuffer class StringBuffer sb = new StringBuffer("Hello"); System.out.println("The given string is: " + sb); //initialize the index and ch values int index = -1; char ch = 'o'; System.out.println("The initialize index and ch values are: " + index + " and " + ch); //using the setCharAt() method sb.setCharAt(index, ch); System.out.println("After setting the character the string is: " + sb); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:-
The given string is: Hello The initialize index and ch values are: -1 and o java.lang.StringIndexOutOfBoundsException: Index -1 out of bounds for length 5 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckIndex(Preconditions.java:106) at java.base/jdk.internal.util.Preconditions.checkIndex(Preconditions.java:302) at java.base/java.lang.String.checkIndex(String.java:4557) at java.base/java.lang.AbstractStringBuilder.setCharAt(AbstractStringBuilder.java:522) at java.base/java.lang.StringBuffer.setCharAt(StringBuffer.java:299) at SetChar.main(SetChar.java:14) Exception: java.lang.StringIndexOutOfBoundsException: Index -1 out of bounds for length 5
示例:在给定索引处设置字符串中的字符时遇到 IndexOutOfBoundsException
如果给定的索引值**大于字符串长度**,此方法会抛出**IndexOutOfBoundsException**。
在以下程序中,我们使用值**“TutorialsPoint”**实例化**StringBuffer 类**。然后,使用**setCharAt()** 方法,我们尝试在指定索引**20**处设置字符**‘J’**,其值大于字符串长度。
public class SetChar { public static void main(String[] args) { try { //instantiate the StringBuffer class StringBuffer sb = new StringBuffer("TutorialsPoint"); System.out.println("The given string is: " + sb); //initialize the index and ch values int index = 20; char ch = 'j'; System.out.println("The initialize index and ch values are: " + index + " and " + ch); //using the setCharAt() method sb.setCharAt(index, ch); System.out.println("After setting the character the string is: " + sb); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
上述程序产生以下结果:-
The given string is: TutorialsPoint The initialize index and ch values are: 20 and j java.lang.StringIndexOutOfBoundsException: Index 20 out of bounds for length 14 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckIndex(Preconditions.java:106) at java.base/jdk.internal.util.Preconditions.checkIndex(Preconditions.java:302) at java.base/java.lang.String.checkIndex(String.java:4557) at java.base/java.lang.AbstractStringBuilder.setCharAt(AbstractStringBuilder.java:522) at java.base/java.lang.StringBuffer.setCharAt(StringBuffer.java:299) at SetChar.main(SetChar.java:14) Exception: java.lang.StringIndexOutOfBoundsException: Index 20 out of bounds for length 14