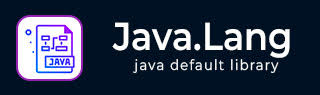
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StringBuffer subSequence() 方法
Java StringBuffer subSequence() 方法用于从 StringBuffer 对象中检索子序列。子序列是字符串或序列的一小部分。
subsequence() 方法接受两个整数参数,分别表示开始和结束索引值。如果开始索引或结束索引值为负数,开始索引值大于结束索引值,以及结束索引值大于序列长度,则会抛出异常。
语法
以下是 Java StringBuffer subSequence() 方法的语法:
public CharSequence subSequence(int start, int end)
参数
- start - 这是开始索引,包含在内。
- end - 这是结束索引,不包含在内。
返回值
此方法返回指定的子序列。
示例:获取 StringBuffer 字符串的子序列
如果开始索引和结束索引值为正数且小于序列长度,则 subsequence() 方法会返回给定序列的子序列。
在以下程序中,我们使用“Java Programming”的值实例化StringBuffer 类。然后,使用subsequence() 方法,我们尝试在指定的开始索引 5和结束索引 16处检索给定序列的子序列。
public class SubSequence { public static void main(String[] args) { //instantiate the StringBuffer class StringBuffer sb = new StringBuffer("Java Programming"); System.out.println("The string is: " + sb.toString()); //initialize the startIndex and endIndex values int startIndex = 5; int endIndex = 16; System.out.println("The initialize values of the startIndex and endIndex are: " + startIndex + " and " + endIndex); //using the subSequence() method System.out.println("The sub-sequence of the given sequence is: " + sb.subSequence(startIndex, endIndex)); } }
输出
执行上述程序后,将产生以下结果:
The string is: Java Programming The initialize values of the startIndex and endIndex are: 5 and 16 The sub-sequence of the given sequence is: Programming
示例:在获取 StringBuffer 字符串的子序列时遇到 StringIndexOutOfBoundsException
如果给定的开始索引和结束索引值为负数,则此方法会抛出StringIndexOutOfBoundsException异常。
在以下程序中,我们使用“Tutorials Point”的值创建一个StringBuffer 类的对象。使用subsequence() 方法,我们尝试在指定的开始索引 -1和结束索引 -2处检索给定序列的子序列。
public class SubSequence { public static void main(String[] args) { try { //create an object of the StringBuffer class StringBuffer sb = new StringBuffer("Tutorials Point"); System.out.println("The string is: " + sb.toString()); //initialize the startIndex and endIndex values int startIndex = -1; int endIndex = -2; System.out.println("The initialize values of the startIndex and endIndex are: " + startIndex + " and " + endIndex); //using the subSequence() method System.out.println("The sub-sequence of the given sequence is: " + sb.subSequence(startIndex, endIndex)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
The string is: Tutorials Point The initialize values of the startIndex and endIndex are: -1 and -2 java.lang.StringIndexOutOfBoundsException: Range [-1, -2) out of bounds for length 15 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckFromToIndex(Preconditions.java:112) at java.base/jdk.internal.util.Preconditions.checkFromToIndex(Preconditions.java:349) at java.base/java.lang.AbstractStringBuilder.substring(AbstractStringBuilder.java:1057) at java.base/java.lang.StringBuffer.subSequence(StringBuffer.java:516) at SubSequence.main(SubSequence.java:15) Exception: java.lang.StringIndexOutOfBoundsException: Range [-1, -2) out of bounds for length 15
示例:在获取 StringBuffer 字符串的子序列时遇到 StringIndexOutOfBoundsException
如果结束索引值大于序列长度,则 subsequence() 方法会抛出StringIndexOutOfBoundsException异常。
在此程序中,我们使用以下值实例化StringBuffer 类
“Hello World”
使用subsequence() 方法,我们尝试在指定的开始索引 5和结束索引 20处检索给定序列的子序列。
public class SubSequence { public static void main(String[] args) { try { //instantiate the StringBuffer class StringBuffer sb = new StringBuffer("Hello World"); System.out.println("The string is: " + sb.toString()); //initialize the startIndex and endIndex values int startIndex = 2; int endIndex = 25; System.out.println("The initialize values of the startIndex and endIndex are: " + startIndex + " and " + endIndex); //using the subSequence() method System.out.println("The sub-sequence of the given sequence is: " + sb.subSequence(startIndex, endIndex)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
上述程序产生以下结果:
The string is: Hello World The initialize values of the startIndex and endIndex are: 2 and 25 java.lang.StringIndexOutOfBoundsException: Range [2, 25) out of bounds for length 11 at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:55) at java.base/jdk.internal.util.Preconditions$1.apply(Preconditions.java:52) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:213) at java.base/jdk.internal.util.Preconditions$4.apply(Preconditions.java:210) at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:98) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckFromToIndex(Preconditions.java:112) at java.base/jdk.internal.util.Preconditions.checkFromToIndex(Preconditions.java:349) at java.base/java.lang.AbstractStringBuilder.substring(AbstractStringBuilder.java:1057) at java.base/java.lang.StringBuffer.subSequence(StringBuffer.java:516) at SubSequence.main(SubSequence.java:15) Exception: java.lang.StringIndexOutOfBoundsException: Range [2, 25) out of bounds for length 11
java_lang_stringbuffer.htm
广告