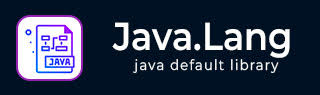
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java System arraycopy() 方法
描述
Java System arraycopy() 方法将数组从指定的源数组(从指定位置开始)复制到目标数组的指定位置。将由src引用的源数组中的一个子序列数组组件复制到由dest引用的目标数组中。复制的组件数等于length参数。
源数组中从srcPos到srcPos + length - 1位置的组件分别复制到目标数组中从destPos到destPos + length - 1位置。
声明
以下是java.lang.System.arraycopy()方法的声明
public static void arraycopy(Object src, int srcPos, Object dest, int destPos, int length)
参数
src − 这是源数组。
srcPos − 这是源数组中的起始位置。
dest − 这是目标数组。
destPos − 这是目标数据中的起始位置。
length − 这是要复制的数组元素的数量。
返回值
此方法不返回值。
异常
IndexOutOfBoundsException − 如果复制会导致访问数组边界之外的数据。
ArrayStoreException − 如果由于类型不匹配,src数组中的元素无法存储到dest数组中。
NullPointerException − 如果src或dest为null。
示例:复制int数组
以下示例显示了 Java System arraycopy() 方法的使用。在此程序中,我们创建了两个int数组并用一些值初始化了它们。现在使用 System.arraycopy() 方法,将第一个数组 arr1 的第一个元素复制到第二个数组的索引 0 处。然后我们打印第二个数组以显示更新后的数组作为结果。
package com.tutorialspoint; public class SystemDemo { public static void main(String[] args) { int arr1[] = { 0, 1, 2, 3, 4, 5 }; int arr2[] = { 5, 10, 20, 30, 40, 50 }; // copies an array from the specified source array System.arraycopy(arr1, 0, arr2, 0, 1); System.out.print("array2 = "); for(int i= 0; i < arr2.length; i++) { System.out.print(arr2[i] + " "); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
array2 = 0 10 20 30 40
示例:复制字符串数组
以下示例显示了 Java System arraycopy() 方法的使用。在此程序中,我们创建了两个字符串数组并用一些值初始化了它们。现在使用 System.arraycopy() 方法,将第一个数组 arr1 的第一个元素复制到第二个数组的索引 0 处。然后我们打印第二个数组以显示更新后的数组作为结果。
package com.tutorialspoint; public class SystemDemo { public static void main(String[] args) { String arr1[] = { "0", "1", "2", "3", "4", "5" }; String arr2[] = { "5", "10", "20", "30", "40", "50" }; // copies an array from the specified source array System.arraycopy(arr1, 0, arr2, 0, 1); System.out.print("array2 = "); for(int i= 0; i < arr2.length; i++) { System.out.print(arr2[i] + " "); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
array2 = 0 10 20 30 40
示例:复制对象数组
以下示例显示了 Java System arraycopy() 方法的使用。在此程序中,我们创建了两个 Student 对象数组并用一些值初始化了它们。现在使用 System.arraycopy() 方法,将第一个数组 arr1 的第一个元素复制到第二个数组的索引 0 处。然后我们打印第二个数组以显示更新后的数组作为结果。
package com.tutorialspoint; public class SystemDemo { public static void main(String[] args) { Student arr1[] = { new Student(1, "Julie") }; Student arr2[] = { new Student(2, "Robert"), new Student(3, "Adam") }; // copies an array from the specified source array System.arraycopy(arr1, 0, arr2, 0, 1); System.out.print("array2 = "); for(int i= 0; i < arr2.length; i++) { System.out.print(arr2[i] + " "); } } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
array2 = [ 1, Julie ] [ 3, Adam ]