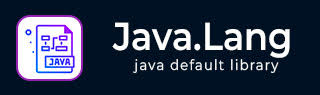
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java Thread activeCount() 方法
描述
Java Thread activeCount() 方法返回当前线程的线程组中活动线程的数量。
声明
以下是 java.lang.Thread.activeCount() 方法的声明
public static int activeCount()
参数
无
返回值
此方法返回当前线程的线程组中活动线程的数量。
异常
无
示例:在单线程程序中获取活动线程计数
以下示例演示了 Java Thread activeCount() 方法的使用。在这个程序中,我们创建了一个类 ThreadDemo。在 main 方法中,使用 currentThread() 方法检索当前线程并打印出来。使用 activeCount(),检索活动线程的计数并打印出来。接下来,我们创建了一个活动线程数组并迭代它们以在控制台上打印。
package com.tutorialspoint; public class ThreadDemo { public static void main(String[] args) { Thread t = Thread.currentThread(); t.setName("Admin Thread"); // set thread priority to 1 t.setPriority(1); // prints the current thread System.out.println("Thread = " + t); int count = Thread.activeCount(); System.out.println("currently active threads = " + count); Thread th[] = new Thread[count]; // returns the number of threads put into the array Thread.enumerate(th); // prints active threads for (int i = 0; i < count; i++) { System.out.println(i + ": " + th[i]); } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
Thread = Thread[Admin Thread,1,main] currently active threads = 1 0: Thread[Admin Thread,1,main]
示例:在多线程程序中获取活动线程计数
以下示例演示了 Java Thread activeCount() 方法的使用。在这个程序中,我们创建了一个线程类 ThreadDemo,它实现了 Runnable 接口。在构造函数中,使用 currentThread() 方法检索当前线程,并使用 new Thread 创建一个新线程。使用 activeCount(),检索活动线程的计数并打印出来。
package com.tutorialspoint; public class ThreadDemo implements Runnable { ThreadDemo() { // main thread Thread currThread = Thread.currentThread(); // thread created Thread t = new Thread(this, "Admin Thread"); // this will call run() function t.start(); int count = Thread.activeCount(); System.out.println("currently active threads = " + count); } public void run() { System.out.println("This is run() method"); } public static void main(String args[]) { new ThreadDemo(); } }
输出
让我们编译并运行以上程序,这将产生以下结果:
This is run() method currently active threads = 2
java_lang_thread.htm
广告