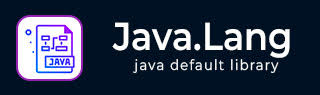
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java Thread dumpStack() 方法
描述
Java Thread dumpStack() 方法将当前线程的堆栈跟踪打印到标准错误流。此方法仅用于调试。
声明
以下是java.lang.Thread.dumpStack() 方法的声明
public static void dumpStack()
参数
无
返回值
此方法不返回任何值。
异常
无
示例:在单线程环境中打印线程堆栈跟踪
以下示例演示了 Java Thread dumpStack() 方法的使用。在这个程序中,我们创建了一个 ThreadDemo 类。在 main 方法中,我们使用 currentThread() 方法检索了当前线程,然后使用 activeCount() 方法打印了活动线程数。最后,我们使用 dumpStack() 方法打印了堆栈跟踪。
package com.tutorialspoint; public class ThreadDemo { public static void main(String[] args) { Thread t = Thread.currentThread(); t.setName("Admin Thread"); // set thread priority to 1 t.setPriority(1); // prints the current thread System.out.println("Thread = " + t); int count = Thread.activeCount(); System.out.println("currently active threads = " + count); /* prints a stack trace of the current thread to the standard error stream, used for debugging */ Thread.dumpStack(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Thread = Thread[#1,Admin Thread,1,main] currently active threads = 1 java.lang.Exception: Stack trace at java.base/java.lang.Thread.dumpStack(Thread.java:2209) at com.tutorialspoint.ThreadDemo.main(ThreadDemo.java:21)
示例:在多线程环境中打印线程堆栈跟踪
以下示例演示了 Java Thread dumpStack() 方法的使用。在这个程序中,我们通过实现 Runnable 接口创建了一个线程类 ThreadDemo。在构造函数中,我们使用 currentThread() 方法检索了当前线程,然后创建了另一个线程并使用 start() 方法启动它。最后,我们使用 dumpStack() 方法打印了堆栈跟踪。在 main 方法中,创建了 ThreadDemo 类的实例。
package com.tutorialspoint; public class ThreadDemo implements Runnable { ThreadDemo() { // main thread Thread currThread = Thread.currentThread(); // thread created Thread t = new Thread(this, "Admin Thread"); System.out.println("current thread = " + currThread); System.out.println("thread created = " + t); // this will call run() function t.start(); /* prints a stack trace of the current thread to the standard error stream, used for debugging */ Thread.dumpStack(); } public void run() { System.out.println("This is run() method"); } public static void main(String args[]) { new ThreadDemo(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
current thread = Thread[#1,main,5,main] thread created = Thread[#21,Admin Thread,5,main] This is run() method java.lang.Exception: Stack trace at java.base/java.lang.Thread.dumpStack(Thread.java:2209) at com.tutorialspoint.ThreadDemo.<init>(ThreadDemo.java:20) at com.tutorialspoint.ThreadDemo.main(ThreadDemo.java:28)
java_lang_thread.htm
广告