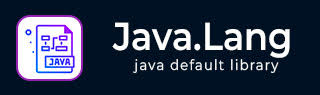
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java Thread getId() 方法
描述
Java Thread getId() 方法返回此线程的标识符。线程 ID 是在创建此线程时生成的正长整数。
线程 ID 是唯一的,在其生命周期内保持不变。当线程终止时,此线程 ID 可能会被重用。
声明
以下是 java.lang.Thread.getId() 方法的声明
public long getId()
参数
无
返回值
此方法返回此线程的 ID。
异常
无
示例:获取使用 Runnable 接口创建的线程的 ID
以下示例演示了 Java Thread getId() 方法的使用。在此程序中,我们通过实现 Runnable 接口创建了一个名为 ThreadDemo 的类。在构造函数中,我们创建了一个新的线程,其默认名称为 Admin Thread 并打印了它。使用 start() 方法启动线程。在 run() 方法中,使用 getId() 打印线程的 ID。在 main 方法中,创建 ThreadDemo 对象。
package com.tutorialspoint; public class ThreadDemo implements Runnable { Thread t; ThreadDemo() { // thread created t = new Thread(this, "Admin Thread"); // set thread priority t.setPriority(1); // print thread created System.out.println("thread = " + t); // this will call run() function t.start(); } public void run() { // returns the name of this Thread. System.out.println("Name = " + t.getName()); // returns the id of this Thread. System.out.println("Id = " + t.getId()); } public static void main(String args[]) { new ThreadDemo(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
thread = Thread[#21,Admin Thread,1,main] Name = Admin Thread Id = 21
示例:获取使用 Thread 类创建的线程的 ID
以下示例演示了 Java Thread getId() 方法的使用。在此程序中,我们通过扩展 Thread 类创建了一个名为 ThreadDemo 的类。在构造函数中,我们创建了一个新的线程,其默认名称为 Admin Thread 并打印了它。使用 start() 方法启动线程。在 run() 方法中,使用 getId() 打印线程的 ID。在 main 方法中,创建 ThreadDemo 对象。
package com.tutorialspoint; public class ThreadDemo extends Thread { Thread t; ThreadDemo() { // thread created t = new Thread(this, "Admin Thread"); // set thread priority t.setPriority(1); // print thread created System.out.println("thread = " + t); // this will call run() function t.start(); } public void run() { // returns the name of this Thread. System.out.println("Name = " + t.getName()); // returns the id of this Thread. System.out.println("Id = " + t.getId()); } public static void main(String args[]) { new ThreadDemo(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
thread = Thread[#22,Admin Thread,1,main] Name = Admin Thread Id = 22
java_lang_thread.htm
广告