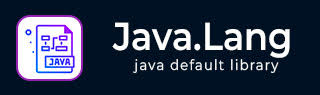
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java Thread join() 方法
描述
Java Thread join(long millis, int nanos) 方法最多等待 millis 毫秒加上 nanos 纳秒,直到该线程结束。
声明
以下是 java.lang.Thread.join() 方法的声明
public final void join(long millis, int nanos) throws InterruptedException
参数
millis - 这是要等待的毫秒数。
nanos - 这是要额外等待的 999999 纳秒。
返回值
此方法不返回值。
异常
IllegalArgumentException - 如果 millis 的值为负数,或者 nanos 的值不在 0-999999 范围内。
InterruptedException - 如果任何线程中断了当前线程。当抛出此异常时,当前线程的中断状态将被清除。
示例:使线程等待
以下示例演示了 Java Thread join() 方法的使用。在此程序中,我们通过实现 Runnable 接口创建了一个线程类 ThreadDemo。在构造函数中,使用 currentThread() 方法获取当前线程。打印其名称,并使用 isAlive() 检查线程是否处于活动状态。
在 main 方法中,我们使用 ThreadDemo 创建了一个线程,并使用 start() 方法启动线程。现在,使用 join() 使线程等待给定的时间段,然后打印线程名称,并再次使用 isAlive() 打印其处于活动状态。
package com.tutorialspoint; public class ThreadDemo implements Runnable { public void run() { Thread t = Thread.currentThread(); System.out.print(t.getName()); //checks if this thread is alive System.out.println(", status = " + t.isAlive()); } public static void main(String args[]) throws Exception { Thread t = new Thread(new ThreadDemo()); // this will call run() function t.start(); /* waits at most 2000 milliseconds plus 500 nanoseconds for this thread to die */ t.join(2000, 500); System.out.println("after waiting for 2000 milliseconds plus 500 nanoseconds ..."); System.out.print(t.getName()); //checks if this thread is alive System.out.println(", status = " + t.isAlive()); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Thread-0, status = true after waiting for 2000 milliseconds plus 500 nanoseconds ... Thread-0, status = false
java_lang_thread.htm
广告