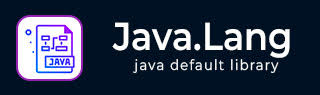
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包补充
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java Thread setPriority() 方法
描述
Java Thread setPriority() 方法更改此线程的优先级。
声明
以下是 java.lang.Thread.setPriority() 方法的声明
public final void setPriority(int newPriority)
参数
newPriority − 这是要设置此线程的优先级。
返回值
此方法不返回任何值。
异常
IllegalArgumentException − 如果优先级不在 MIN_PRIORITY 到 MAX_PRIORITY 范围内。
SecurityException − 如果当前线程无法修改此线程。
示例:设置线程的优先级
以下示例演示了 Java Thread setPriority() 方法的用法。在这个示例中,我们使用 main 方法中的 activeThread() 方法检索当前活动线程,并使用 setPriority() 方法将优先级设置为 1,然后打印带有活动计数的线程。
package com.tutorialspoint; public class ThreadDemo { public static void main(String[] args) { Thread t = Thread.currentThread(); t.setName("Admin Thread"); // set thread priority to 1 t.setPriority(1); // prints the current thread System.out.println("Thread = " + t); int count = Thread.activeCount(); System.out.println("currently active threads = " + count); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Thread = Thread[Admin Thread,1,main] currently active threads = 1
示例:设置线程的最高优先级
以下示例演示了 Java Thread setPriority() 方法的用法。在这个示例中,我们使用 main 方法中的 activeThread() 方法检索当前活动线程,并使用 setPriority() 方法将优先级设置为最高,然后打印带有活动计数的线程。
package com.tutorialspoint; public class ThreadDemo { public static void main(String[] args) { Thread t = Thread.currentThread(); t.setName("Admin Thread"); // set thread priority to MAX_PRIORITY t.setPriority(Thread.MAX_PRIORITY); // prints the current thread System.out.println("Thread = " + t); int count = Thread.activeCount(); System.out.println("currently active threads = " + count); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Thread = Thread[Admin Thread,1,main] currently active threads = 1
示例:设置线程的最低优先级
以下示例演示了 Java Thread setPriority() 方法的用法。在这个示例中,我们使用 main 方法中的 activeThread() 方法检索当前活动线程,并使用 setPriority() 方法将优先级设置为最低,然后打印带有活动计数的线程。
package com.tutorialspoint; public class ThreadDemo { public static void main(String[] args) { Thread t = Thread.currentThread(); t.setName("Admin Thread"); // set thread priority to MIN_PRIORITY t.setPriority(Thread.MIN_PRIORITY); // prints the current thread System.out.println("Thread = " + t); int count = Thread.activeCount(); System.out.println("currently active threads = " + count); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Thread = Thread[Admin Thread,1,main] currently active threads = 1
java_lang_thread.htm
广告