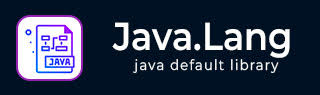
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java Thread sleep() 方法
描述
Java Thread sleep(long millis) 方法使当前正在执行的线程休眠指定毫秒数,受系统计时器和调度程序的精度和准确性的影响。
声明
以下是java.lang.Thread.sleep() 方法的声明
public static void sleep(long millis) throws InterruptedException
参数
millis − 这是休眠的毫秒数。
返回值
此方法不返回值。
异常
InterruptedException − 如果任何线程已中断当前线程。当抛出此异常时,当前线程的中断状态将被清除。
示例:在多线程环境中使用 sleep 用于线程
以下示例演示了 Java Thread sleep() 方法的使用。在这个程序中,我们通过实现 Runnable 接口创建了一个线程类 ThreadDemo。在 run 方法中,一个 for 循环正在运行,我们调用 sleep() 方法来创建给定的延迟。在 main() 方法中,创建并启动两个 ThreadDemo 类的线程以打印结果。
package com.tutorialspoint; public class ThreadDemo implements Runnable { Thread t; public void run() { for (int i = 10; i < 13; i++) { System.out.println(Thread.currentThread().getName() + " " + i); try { // thread to sleep for 1000 milliseconds Thread.sleep(1000); } catch (Exception e) { System.out.println(e); } } } public static void main(String[] args) throws Exception { Thread t = new Thread(new ThreadDemo()); // this will call run() function t.start(); Thread t2 = new Thread(new ThreadDemo()); // this will call run() function t2.start(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Thread-0 10 Thread-1 10 Thread-0 11 Thread-1 11 Thread-0 12 Thread-1 12
示例:在单线程环境中使用 sleep 用于线程
以下示例演示了 Java Thread sleep() 方法的使用。在这个程序中,我们创建了一个类 ThreadDemo。在 main 方法中,使用 currentThread() 方法检索当前线程,并使用 sleep() 引入给定时间的延迟,然后打印线程。
package com.tutorialspoint; public class ThreadDemo { public static void main(String[] args) { Thread t = Thread.currentThread(); t.setName("Admin Thread"); // set thread priority to 1 t.setPriority(1); try { // thread to sleep for 1000 milliseconds Thread.sleep(1000); } catch (Exception e) { System.out.println(e); } // prints the current thread System.out.println("Thread = " + t); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Thread = Thread[Admin Thread,1,main]
java_lang_thread.htm
广告