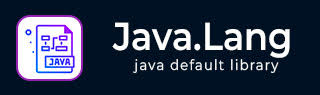
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - ThreadGroup activeCount() 方法
描述
Java ThreadGroup activeCount() 方法返回此线程组中活动线程数量的估计值。
声明
以下是 java.lang.ThreadGroup.activeCount() 方法的声明
public int activeCount()
参数
无
返回值
此方法返回此线程组以及任何其他以该线程组为祖先的线程组中活动线程数量的估计值。
异常
无
获取 ThreadGroup 中线程的活动数量示例
以下示例展示了在单个 ThreadGroup 对象的情况下使用 ThreadGroup activeCount() 方法的情况。我们创建了一个 ThreadGroup 对象并为其分配了一个名称。然后,我们使用前面创建的 ThreadGroup 对象创建了两个线程。使用 activeCount() 方法,我们打印活动线程数。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a ThreadGroup ThreadGroup threadGroup = new ThreadGroup("ThreadGroup"); // create a thread Thread t1 = new Thread(threadGroup, this); System.out.println("Starting " + t1.getName() + "..."); t1.start(); // create another thread Thread t2 = new Thread(threadGroup, this); System.out.println("Starting " + t2.getName() + "..."); t2.start(); // display the number of active threads System.out.println("Active threads in \"" + threadGroup.getName() + "\" = " + threadGroup.activeCount()); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } // implements run() public void run() { for(int i = 0; i < 4;i++) { i++; try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println(Thread.currentThread().getName() + " finished executing."); } }
让我们编译并运行上述程序,这将产生以下结果:
Starting Thread-0... Starting Thread-1... Active threads in "ThreadGroup" = 2 Thread-0 finished executing. Thread-1 finished executing.
获取多个 ThreadGroup 对象中线程的活动数量示例
以下示例展示了在多个 ThreadGroup 对象的情况下使用 ThreadGroup activeCount() 方法的情况。我们创建了一个 ThreadGroup 对象并为其分配了一个名称。接下来,我们创建了一个子 ThreadGroup 对象。然后,我们使用前面创建的 ThreadGroup 对象创建了两个线程。使用 activeCount() 方法,我们打印父 ThreadGroup 对象和子 ThreadGroup 对象中的活动线程数。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a parent ThreadGroup ThreadGroup pThreadGroup = new ThreadGroup("Parent ThreadGroup"); // create a child ThreadGroup for parent ThreadGroup ThreadGroup cThreadGroup = new ThreadGroup(pThreadGroup, "Child ThreadGroup"); // create a thread Thread t1 = new Thread(pThreadGroup, this); System.out.println("Starting " + t1.getName() + "..."); t1.start(); // create another thread Thread t2 = new Thread(cThreadGroup, this); System.out.println("Starting " + t2.getName() + "..."); t2.start(); // display the number of active threads System.out.println("Active threads in \"" + pThreadGroup.getName() + "\" = " + pThreadGroup.activeCount()); System.out.println("Active threads in \"" + cThreadGroup.getName() + "\" = " + cThreadGroup.activeCount()); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } // implements run() public void run() { for(int i = 0; i < 4;i++) { i++; try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println(Thread.currentThread().getName() + " finished executing."); } }
让我们编译并运行上述程序,这将产生以下结果:
Starting Thread-0... Starting Thread-1... Active threads in "Parent ThreadGroup" = 2 Active threads in "Child ThreadGroup" = 1 Thread-0 finished executing. Thread-1 finished executing.
获取子 ThreadGroup 中线程的活动数量示例
以下示例展示了在子 ThreadGroup 对象的情况下使用 ThreadGroup activeCount() 方法的情况。我们创建了一个 ThreadGroup 对象并为其分配了一个名称。接下来,我们创建了一个子 ThreadGroup 对象。然后,我们使用前面创建的子 ThreadGroup 对象创建了两个线程。使用 activeCount() 方法,我们打印父 ThreadGroup 对象和子 ThreadGroup 对象中的活动线程数。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a parent ThreadGroup ThreadGroup pThreadGroup = new ThreadGroup("Parent ThreadGroup"); // create a child ThreadGroup for parent ThreadGroup ThreadGroup cThreadGroup = new ThreadGroup(pThreadGroup, "Child ThreadGroup"); // create a thread Thread t1 = new Thread(cThreadGroup, this); System.out.println("Starting " + t1.getName() + "..."); t1.start(); // create another thread Thread t2 = new Thread(cThreadGroup, this); System.out.println("Starting " + t2.getName() + "..."); t2.start(); // display the number of active threads System.out.println("Active threads in \"" + pThreadGroup.getName() + "\" = " + pThreadGroup.activeCount()); System.out.println("Active threads in \"" + cThreadGroup.getName() + "\" = " + cThreadGroup.activeCount()); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } // implements run() public void run() { for(int i = 0; i < 4;i++) { i++; try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println(Thread.currentThread().getName() + " finished executing."); } }
让我们编译并运行上述程序,这将产生以下结果:
Starting Thread-0... Starting Thread-1... Active threads in "Parent ThreadGroup" = 2 Active threads in "Child ThreadGroup" = 2 Thread-1 finished executing. Thread-0 finished executing.