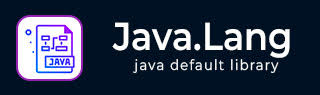
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - ThreadGroup isDestroyed() 方法
描述
Java ThreadGroup isDestroyed() 方法测试此线程组是否已被销毁。
声明
以下是java.lang.ThreadGroup.isDestroyed() 方法的声明
public boolean isDestroyed()
参数
无
返回值
如果此对象已被销毁,则此方法返回 true。
异常
无
检查 ThreadGroup 是否已销毁的示例
以下示例展示了在单个 ThreadGroup 对象的情况下使用 ThreadGroup isDestroyed() 方法的情况。我们创建了一个 ThreadGroup 对象并为其分配了一个名称。然后,我们使用前面创建的 threadgroup 对象创建了两个线程。使用 isDestroyed() 方法,我们获取此 threadgroup 对象的父对象的的状态。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a ThreadGroup ThreadGroup threadGroup = new ThreadGroup("ThreadGroup"); // create a thread Thread t1 = new Thread(threadGroup, this); System.out.println("Starting " + t1.getName() + "..."); t1.start(); // create another thread Thread t2 = new Thread(threadGroup, this); System.out.println("Starting " + t2.getName() + "..."); t2.start(); // returns the status of thread group boolean isDestroyed = threadGroup.isDestroyed(); System.out.println("Status of the threadGroup = " + isDestroyed); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } // implements run() public void run() { for(int i = 0; i < 4;i++) { i++; try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println(Thread.currentThread().getName() + " finished executing."); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Starting Thread-0... Starting Thread-1... Status of the threadGroup = false Thread-1 finished executing. Thread-0 finished executing.
在多个 ThreadGroup 中检查 ThreadGroup 是否已销毁的示例
以下示例展示了在多个 ThreadGroup 对象的情况下使用 ThreadGroup isDestroyed() 方法的情况。我们创建了一个 ThreadGroup 对象并为其分配了一个名称。接下来,我们创建了一个子 ThreadGroup 对象。然后,我们使用前面创建的 threadgroup 对象创建了两个线程。使用 isDestroyed() 方法,我们打印每个 threadgroup 对象的状态。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a parent ThreadGroup ThreadGroup pThreadGroup = new ThreadGroup("parent ThreadGroup"); // create a child ThreadGroup for parent ThreadGroup ThreadGroup cThreadGroup = new ThreadGroup(pThreadGroup, "child ThreadGroup"); // create a thread Thread t1 = new Thread(pThreadGroup, this); System.out.println("Starting " + t1.getName() + "..."); t1.start(); // create another thread Thread t2 = new Thread(cThreadGroup, this); System.out.println("Starting " + t2.getName() + "..."); t2.start(); // returns the status of thread group System.out.println("Status of pThreadGroup = " + pThreadGroup.isDestroyed()); System.out.println("Status of cThreadGroup = " + cThreadGroup.isDestroyed()); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } // implements run() public void run() { for(int i = 0; i < 4;i++) { i++; try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println(Thread.currentThread().getName() + " finished executing."); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Starting Thread-0... Starting Thread-1... Status of pThreadGroup = false Status of cThreadGroup = false Thread-1 finished executing. Thread-0 finished executing.
在子线程组和孙线程组的情况下检查 ThreadGroup 是否已销毁的示例
以下示例展示了在子线程组和孙线程组的情况下使用 ThreadGroup isDestroyed() 方法的情况。我们创建了一个 ThreadGroup 对象并为其分配了一个名称。接下来,我们创建了一个子 ThreadGroup 对象。然后,我们使用前面创建的子线程组和孙线程组对象创建了两个线程。使用 isDestroyed() 方法,我们打印每个 threadgroup 对象的状态。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a parent ThreadGroup ThreadGroup pThreadGroup = new ThreadGroup("Parent ThreadGroup"); // create a child ThreadGroup for parent ThreadGroup ThreadGroup cThreadGroup = new ThreadGroup(pThreadGroup, "Child ThreadGroup"); // create a grandchild ThreadGroup for parent ThreadGroup ThreadGroup gThreadGroup = new ThreadGroup(cThreadGroup, "GrandChild ThreadGroup"); // create a thread Thread t1 = new Thread(cThreadGroup, this); System.out.println("Starting " + t1.getName() + "..."); t1.start(); // create another thread Thread t2 = new Thread(gThreadGroup, this); System.out.println("Starting " + t2.getName() + "..."); t2.start(); // returns the status of thread group System.out.println("Status of pThreadGroup = " + pThreadGroup.isDestroyed()); System.out.println("Status of cThreadGroup = " + cThreadGroup.isDestroyed()); System.out.println("Status of gThreadGroup = " + gThreadGroup.isDestroyed()); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } // implements run() public void run() { for(int i = 0; i < 4;i++) { i++; try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println(Thread.currentThread().getName() + " finished executing."); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Starting Thread-0... Starting Thread-1... Status of pThreadGroup = false Status of cThreadGroup = false Status of gThreadGroup = false Thread-0 finished executing. Thread-1 finished executing.