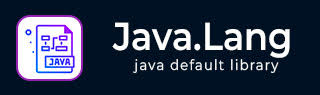
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 实用资源
- Java.lang - 讨论
Java - ThreadGroup toString() 方法
描述
Java ThreadGroup toString() 方法返回此线程组的字符串表示形式。
声明
以下是 java.lang.ThreadGroup.toString() 方法的声明
public String toString()
参数
无
返回值
此方法返回此线程组的字符串表示形式。
异常
无
获取 ThreadGroup 的字符串表示形式示例
以下示例演示了在单个 ThreadGroup 对象的情况下使用 ThreadGroup toString() 方法。我们创建了一个 ThreadGroup 对象并为其分配了一个名称。然后,我们使用前面创建的线程组对象创建了两个线程。使用 toString() 方法,我们获取了线程组对象的字符串表示形式。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a ThreadGroup ThreadGroup threadGroup = new ThreadGroup("ThreadGroup"); // create a thread Thread t1 = new Thread(threadGroup, this); System.out.println("Starting " + t1.toString() + "..."); t1.start(); // create another thread Thread t2 = new Thread(threadGroup, this); System.out.println("Starting " + t2.toString() + "..."); t2.start(); // returns the string representation of thread group System.out.println("String representation of threadGroup = " + threadGroup.toString()); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } // implements run() public void run() { for(int i = 0; i < 4;i++) { i++; try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println(Thread.currentThread().toString() + " finished executing."); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Starting Thread[Thread-0,5,ThreadGroup]... Starting Thread[Thread-1,5,ThreadGroup]... String representation of threadGroup = java.lang.ThreadGroup[name=ThreadGroup,maxpri=10] Thread[Thread-1,5,ThreadGroup] finished executing. Thread[Thread-0,5,ThreadGroup] finished executing.
获取多个 ThreadGroup 对象的字符串表示形式示例
以下示例演示了在多个 ThreadGroup 对象的情况下使用 ThreadGroup toString() 方法。我们创建了一个 ThreadGroup 对象并为其分配了一个名称。接下来,我们创建了一个子 ThreadGroup 对象。然后,我们使用前面创建的线程组对象创建了两个线程。使用 toString() 方法,我们打印了每个线程组对象的字符串表示形式。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a parent ThreadGroup ThreadGroup pThreadGroup = new ThreadGroup("parent ThreadGroup"); // create a child ThreadGroup for parent ThreadGroup ThreadGroup cThreadGroup = new ThreadGroup(pThreadGroup, "child ThreadGroup"); // create a thread Thread t1 = new Thread(pThreadGroup, this); System.out.println("Starting " + t1.toString() + "..."); t1.start(); // create another thread Thread t2 = new Thread(cThreadGroup, this); System.out.println("Starting " + t2.toString() + "..."); t2.start(); // returns the string representation of thread groups System.out.println("String representation of pThreadGroup = " + pThreadGroup.toString()); System.out.println("String representation of cThreadGroup = " + cThreadGroup.toString()); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } // implements run() public void run() { for(int i = 0; i < 4;i++) { i++; try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println(Thread.currentThread().toString() + " finished executing."); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Starting Thread[Thread-0,5,parent ThreadGroup]... Starting Thread[Thread-1,5,child ThreadGroup]... String representation of pThreadGroup = java.lang.ThreadGroup[name=parent ThreadGroup,maxpri=10] String representation of cThreadGroup = java.lang.ThreadGroup[name=child ThreadGroup,maxpri=10] Thread[Thread-1,5,child ThreadGroup] finished executing. Thread[Thread-0,5,parent ThreadGroup] finished executing.
获取子/孙 ThreadGroup 对象的字符串表示形式示例
以下示例演示了在子线程组和孙线程组对象的情况下使用 ThreadGroup toString() 方法。我们创建了一个 ThreadGroup 对象并为其分配了一个名称。接下来,我们创建了一个子 ThreadGroup 对象。然后,我们使用前面创建的子线程组和孙线程组对象创建了两个线程。使用 toString() 方法,我们打印了每个线程组对象的字符串表示形式。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a parent ThreadGroup ThreadGroup pThreadGroup = new ThreadGroup("Parent ThreadGroup"); // create a child ThreadGroup for parent ThreadGroup ThreadGroup cThreadGroup = new ThreadGroup(pThreadGroup, "Child ThreadGroup"); // create a grandchild ThreadGroup for parent ThreadGroup ThreadGroup gThreadGroup = new ThreadGroup(cThreadGroup, "GrandChild ThreadGroup"); // create a thread Thread t1 = new Thread(cThreadGroup, this); System.out.println("Starting " + t1.toString() + "..."); t1.start(); // create another thread Thread t2 = new Thread(gThreadGroup, this); System.out.println("Starting " + t2.toString() + "..."); t2.start(); // returns the string representation of thread groups System.out.println("String representation of pThreadGroup = " + pThreadGroup.toString()); System.out.println("String representation of cThreadGroup = " + cThreadGroup.toString()); System.out.println("String representation of gThreadGroup = " + gThreadGroup.toString()); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } // implements run() public void run() { for(int i = 0; i < 4;i++) { i++; try { Thread.sleep(50); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println(Thread.currentThread().toString() + " finished executing."); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Starting Thread[Thread-0,5,Child ThreadGroup]... Starting Thread[Thread-1,5,GrandChild ThreadGroup]... String representation of pThreadGroup = java.lang.ThreadGroup[name=Parent ThreadGroup,maxpri=10] String representation of cThreadGroup = java.lang.ThreadGroup[name=Child ThreadGroup,maxpri=10] String representation of gThreadGroup = java.lang.ThreadGroup[name=GrandChild ThreadGroup,maxpri=10] Thread[Thread-0,5,Child ThreadGroup] finished executing. Thread[Thread-1,5,GrandChild ThreadGroup] finished executing.
java_lang_threadgroup.htm
广告