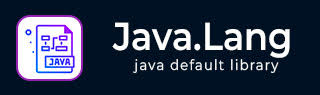
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - ThreadGroup uncaughtException() 方法
描述
当此线程组中的线程由于未捕获的异常而停止,并且该线程未安装特定的 Thread.UncaughtExceptionHandler 时,Java ThreadGroup uncaughtException() 方法由 Java 虚拟机调用。
声明
以下是java.lang.ThreadGroup.uncaughtException() 方法的声明
public void uncaughtException(Thread t, Throwable e)
参数
t − 即将退出的线程。
e − 未捕获的异常。
返回值
此方法不返回任何值。
异常
无
ThreadGroup 对象中检查未处理异常的示例
以下示例演示了在 ThreadGroup 对象的情况下使用 ThreadGroup uncaughtException() 方法。我们创建了一个新类 NewThreadGroup,它扩展了 ThreadGroup。它具有 uncaughtException() 方法的重写实现。使用 NewThreadGroup,我们正在创建一个线程组对象。然后,我们使用前面创建的子线程组和孙线程组对象创建了两个线程。启动线程后,我们使用 interrupt() 方法调用来中断流程。处理 InterruptedException 后,我们将抛出一个 RuntimeException,最终由 uncaughtException() 方法处理。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a ThreadGroup object NewThreadGroup threadGroup = new NewThreadGroup("ThreadGroup"); // create a thread Thread t1 = new Thread(threadGroup, this); System.out.println("Starting " + t1.toString() + "..."); t1.start(); // create another thread Thread t2 = new Thread(threadGroup, this); System.out.println("Starting " + t2.toString() + "..."); t2.start(); try { Thread.sleep(500); } catch(InterruptedException ex) {} // interrupt the two threads t1.interrupt(); t2.interrupt(); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } public void run() { try { System.out.print(Thread.currentThread().getName()); System.out.println(" executing..."); while(true) { Thread.sleep(500); } } catch(InterruptedException e) { Thread currThread = Thread.currentThread(); System.out.print(currThread.getName()); System.out.println(" interrupted:" + e.toString()); // rethrow the exception throw new RuntimeException(e.getMessage()); } } } class NewThreadGroup extends ThreadGroup { NewThreadGroup(String n) { super(n); } NewThreadGroup(ThreadGroup parent, String n) { super(parent, n); } public void uncaughtException(Thread t, Throwable e) { System.out.println(t + " has unhandled exception:" + e); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Starting Thread[Thread-0,5,ThreadGroup]... Starting Thread[Thread-1,5,ThreadGroup]... Thread-0 executing... Thread-1 executing... Thread-1Thread-0 interrupted:java.lang.InterruptedException: sleep interrupted interrupted:java.lang.InterruptedException: sleep interrupted Thread[Thread-0,5,ThreadGroup] has unhandled exception:java.lang.RuntimeException: sleep interrupted Thread[Thread-1,5,ThreadGroup] has unhandled exception:java.lang.RuntimeException: sleep interrupted
子 ThreadGroup 对象中检查未处理异常的示例
以下示例演示了在父和子 ThreadGroup 对象的情况下使用 ThreadGroup uncaughtException() 方法。我们创建了一个新类 NewThreadGroup,它扩展了 ThreadGroup。它具有 uncaughtException() 方法的重写实现。使用 NewThreadGroup,我们正在创建父、子线程组对象。然后,我们使用前面创建的子线程组和孙线程组对象创建了两个线程。启动线程后,我们使用 interrupt() 方法调用来中断流程。处理 InterruptedException 后,我们将抛出一个 RuntimeException,最终由 uncaughtException() 方法处理。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a parent ThreadGroup NewThreadGroup pThreadGroup = new NewThreadGroup("Parent ThreadGroup"); // create a child ThreadGroup for parent ThreadGroup NewThreadGroup cThreadGroup = new NewThreadGroup(pThreadGroup, "Child ThreadGroup"); // create a thread Thread t1 = new Thread(pThreadGroup, this); System.out.println("Starting " + t1.toString() + "..."); t1.start(); // create another thread Thread t2 = new Thread(cThreadGroup, this); System.out.println("Starting " + t2.toString() + "..."); t2.start(); try { Thread.sleep(500); } catch(InterruptedException ex) {} // interrupt the two threads t1.interrupt(); t2.interrupt(); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } public void run() { try { System.out.print(Thread.currentThread().getName()); System.out.println(" executing..."); while(true) { Thread.sleep(500); } } catch(InterruptedException e) { Thread currThread = Thread.currentThread(); System.out.print(currThread.getName()); System.out.println(" interrupted:" + e.toString()); // rethrow the exception throw new RuntimeException(e.getMessage()); } } } class NewThreadGroup extends ThreadGroup { NewThreadGroup(String n) { super(n); } NewThreadGroup(ThreadGroup parent, String n) { super(parent, n); } public void uncaughtException(Thread t, Throwable e) { System.out.println(t + " has unhandled exception:" + e); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Starting Thread[Thread-0,5,Parent ThreadGroup]... Starting Thread[Thread-1,5,Child ThreadGroup]... Thread-0 executing... Thread-1 executing... Thread-1Thread-0 interrupted:java.lang.InterruptedException: sleep interrupted interrupted:java.lang.InterruptedException: sleep interrupted Thread[Thread-0,5,Parent ThreadGroup] has unhandled exception:java.lang.RuntimeException: sleep interrupted Thread[Thread-1,5,Child ThreadGroup] has unhandled exception:java.lang.RuntimeException: sleep interrupted
子线程组和孙线程组对象中检查未处理异常的示例
以下示例演示了在子线程组和孙线程组对象的情况下使用 ThreadGroup uncaughtException() 方法。我们创建了一个新类 NewThreadGroup,它扩展了 ThreadGroup。它具有 uncaughtException() 方法的重写实现。使用 NewThreadGroup,我们正在创建父、子线程组和孙线程组对象。然后,我们使用前面创建的子线程组和孙线程组对象创建了两个线程。启动线程后,我们使用 interrupt() 方法调用来中断流程。处理 InterruptedException 后,我们将抛出一个 RuntimeException,最终由 uncaughtException() 方法处理。
package com.tutorialspoint; public class ThreadGroupDemo implements Runnable { public static void main(String[] args) { ThreadGroupDemo tg = new ThreadGroupDemo(); tg.start(); } public void start() { try { // create a parent ThreadGroup NewThreadGroup pThreadGroup = new NewThreadGroup("Parent ThreadGroup"); // create a child ThreadGroup for parent ThreadGroup NewThreadGroup cThreadGroup = new NewThreadGroup(pThreadGroup, "Child ThreadGroup"); // create a grandchild ThreadGroup for parent ThreadGroup NewThreadGroup gThreadGroup = new NewThreadGroup(cThreadGroup, "GrandChild ThreadGroup"); // create a thread Thread t1 = new Thread(cThreadGroup, this); System.out.println("Starting " + t1.toString() + "..."); t1.start(); // create another thread Thread t2 = new Thread(gThreadGroup, this); System.out.println("Starting " + t2.toString() + "..."); t2.start(); try { Thread.sleep(500); } catch(InterruptedException ex) {} // interrupt the two threads t1.interrupt(); t2.interrupt(); // block until the other threads finish t1.join(); t2.join(); } catch (InterruptedException ex) { System.out.println(ex.toString()); } } public void run() { try { System.out.print(Thread.currentThread().getName()); System.out.println(" executing..."); while(true) { Thread.sleep(500); } } catch(InterruptedException e) { Thread currThread = Thread.currentThread(); System.out.print(currThread.getName()); System.out.println(" interrupted:" + e.toString()); // rethrow the exception throw new RuntimeException(e.getMessage()); } } } class NewThreadGroup extends ThreadGroup { NewThreadGroup(String n) { super(n); } NewThreadGroup(ThreadGroup parent, String n) { super(parent, n); } public void uncaughtException(Thread t, Throwable e) { System.out.println(t + " has unhandled exception:" + e); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Starting Thread[Thread-0,5,Child ThreadGroup]... Starting Thread[Thread-1,5,GrandChild ThreadGroup]... Thread-0 executing... Thread-1 executing... Thread-1Thread-0 interrupted:java.lang.InterruptedException: sleep interrupted interrupted:java.lang.InterruptedException: sleep interrupted Thread[Thread-1,5,GrandChild ThreadGroup] has unhandled exception:java.lang.RuntimeException: sleep interrupted Thread[Thread-0,5,Child ThreadGroup] has unhandled exception:java.lang.RuntimeException: sleep interrupted