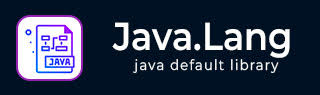
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包补充
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java ThreadLocal initialValue() 方法
描述
Java ThreadLocal initialValue() 方法返回此线程局部变量的当前线程的初始值。
声明
以下是java.lang.ThreadLocal.initialValue() 方法的声明
protected T initialValue()
Learn Java in-depth with real-world projects through our Java certification course. Enroll and become a certified expert to boost your career.
参数
无
返回值
此方法返回此线程局部的初始值。
异常
无
示例:使用 ThreadLocal 对象的初始值作为整数
以下示例演示了 Java ThreadLocal initialValue() 方法的用法。我们通过扩展 Thread 创建了一个 NewThread 类。在这个类中,我们有一个 ThreadLocal 对象 t,其重写了 initialValue() 方法,该方法返回实例值并将其减 1。在 run 方法中,我们打印当前线程名称和实例值。
package com.tutorialspoint; public class ThreadLocalDemo { public static void main (String [] args) { NewThread t1 = new NewThread("R"); NewThread t2 = new NewThread("S"); // this will call run() method t1.start(); t2.start(); } } class NewThread extends Thread { private static ThreadLocal t = new ThreadLocal() { protected Object initialValue() { return Integer.valueOf(n--); } }; private static int n = 10; NewThread(String name) { super(name); } public void run() { for(int i = 0; i < 2; i++){ System.out.println (getName() + " " + t.get()); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
R 9 R 9 S 10 S 10
示例:使用 ThreadLocal 对象的初始值作为双精度浮点数
以下示例演示了 Java ThreadLocal initialValue() 方法的用法。我们通过扩展 Thread 创建了一个 NewThread 类。在这个类中,我们有一个 ThreadLocal 对象 t,其重写了 initialValue() 方法,该方法返回实例值并将其减 1。在 run 方法中,我们打印当前线程名称和实例值。
package com.tutorialspoint; public class ThreadLocalDemo { public static void main (String [] args) { NewThread t1 = new NewThread("R"); NewThread t2 = new NewThread("S"); // this will call run() method t1.start(); t2.start(); } } class NewThread extends Thread { private static ThreadLocal t = new ThreadLocal() { protected Object initialValue() { return Double.valueOf(n--); } }; private static double n = 10.0; NewThread(String name) { super(name); } public void run() { for(int i = 0; i < 2; i++){ System.out.println (getName() + " " + t.get()); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
S 10.0 R 10.0 S 10.0 R 10.0
示例:使用 ThreadLocal 对象的初始值作为单精度浮点数
以下示例演示了 Java ThreadLocal initialValue() 方法的用法。我们通过扩展 Thread 创建了一个 NewThread 类。在这个类中,我们有一个 ThreadLocal 对象 t,其重写了 initialValue() 方法,该方法返回实例值并将其减 1。在 run 方法中,我们打印当前线程名称和实例值。
package com.tutorialspoint; public class ThreadLocalDemo { public static void main (String [] args) { NewThread t1 = new NewThread("R"); NewThread t2 = new NewThread("S"); // this will call run() method t1.start(); t2.start(); } } class NewThread extends Thread { private static ThreadLocal t = new ThreadLocal() { protected Object initialValue() { return Float.valueOf(n--); } }; private static float n = 10.0f; NewThread(String name) { super(name); } public void run() { for(int i = 0; i < 2; i++){ System.out.println (getName() + " " + t.get()); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
R 10.0 R 10.0 S 9.0 S 9.0