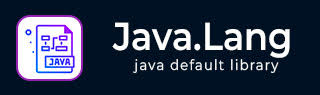
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java Throwable fillInStackTrace() 方法
描述
Java Throwable fillInStackTrace() 方法填充执行堆栈跟踪。此方法在此 Throwable 对象中记录有关当前线程的堆栈帧当前状态的信息。
声明
以下是 java.lang.Throwable.fillInStackTrace() 方法的声明
public Throwable fillInStackTrace()
参数
无
返回值
此方法返回对此 Throwable 实例的引用。
异常
无
示例:打印异常的堆栈跟踪
以下示例显示了 Java Throwable fillInStackTrace() 方法的使用。我们定义了两个方法 function1(),它抛出一个异常,以及 function2(),它调用 function1() 并处理异常,并使用 fillInStackTrace() 方法抛出已处理异常的引用。在 main 方法中,调用 function2() 方法,并在 catch 块中检索并打印异常堆栈跟踪。
package com.tutorialspoint; public class ThrowableDemo { public static void function1() throws Exception { throw new Exception("this is thrown from function1()"); } public static void function2() throws Throwable { try { function1(); } catch(Exception e) { System.err.println("Inside function2():"); e.printStackTrace(); throw e.fillInStackTrace(); } } public static void main(String[] args) throws Throwable { try { function2(); } catch (Exception e) { System.err.println("Caught Inside Main:"); e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Inside function2(): java.lang.Exception: this is thrown from function1() at ThrowableDemo.function1(ThrowableDemo.java:4) at ThrowableDemo.function2(ThrowableDemo.java:9) at ThrowableDemo.main(ThrowableDemo.java:19) Caught Inside Main: java.lang.Exception: this is thrown from function1() at ThrowableDemo.function2(ThrowableDemo.java:13) at ThrowableDemo.main(ThrowableDemo.java:19)
示例:打印 Throwable 的堆栈跟踪
以下示例显示了 Java Throwable fillInStackTrace() 方法的使用。我们定义了一个方法 raiseException(),它在设置堆栈跟踪后抛出一个 Throwable。在 main 方法中,调用 raiseException() 方法,并在 catch 块中使用 fillInStackTrace() 方法检索并打印异常堆栈跟踪。
package com.tutorialspoint; public class ThrowableDemo { public static void main(String[] args) { try { raiseException(); } catch(Throwable e) { // prints stacktrace for this Throwable Object throw e.fillInStackTrace(); } } public static void raiseException() throws Throwable { Throwable t = new Throwable("This is new Exception..."); StackTraceElement[] trace = new StackTraceElement[] { new StackTraceElement("ClassName","methodName","fileName",5) }; // sets the stack trace elements t.setStackTrace(trace); throw t; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Exception in thread "main" java.lang.Throwable: This is new Exception... at com.tutorialspoint.ThrowableDemo.main(ThrowableDemo.java:11)
示例:打印异常的堆栈跟踪
以下示例显示了 Java Throwable fillInStackTrace() 方法的使用。我们定义了一个方法 raiseException(),它在设置堆栈跟踪后抛出一个异常。在 main 方法中,调用 raiseException() 方法,并在 catch 块中使用 fillInStackTrace() 方法检索并打印异常堆栈跟踪。
package com.tutorialspoint; public class ThrowableDemo { public static void main(String[] args) { try { raiseException(); } catch(Throwable e) { // prints stacktrace for this Throwable Object throw e.fillInStackTrace(); } } public static void raiseException() throws Exception { Exception t = new Exception("This is new Exception..."); StackTraceElement[] trace = new StackTraceElement[] { new StackTraceElement("ClassName","methodName","fileName",5) }; // sets the stack trace elements t.setStackTrace(trace); throw t; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Exception in thread "main" java.lang.Exception: This is new Exception... at com.tutorialspoint.ThrowableDemo.main(ThrowableDemo.java:11)