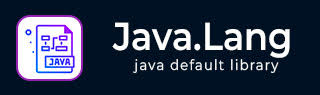
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java Throwable getCause() 方法
描述
Java Throwable getCause() 方法返回此可抛出对象的根本原因,如果原因不存在或未知,则返回 null。
声明
以下是 java.lang.Throwable.getCause() 方法的声明:
public Throwable getCause()
参数
无
返回值
此方法返回此可抛出对象的根本原因,如果原因不存在或未知,则返回 null。
异常
无
示例:获取 Throwable 的初始原因
以下示例演示了 java.lang.Throwable.getCause() 方法的使用。在此程序中,我们定义了一个名为 raiseException() 的方法,在其中定义了一个 Throwable,并使用 initCause() 方法将其初始原因设置为带有消息“ABCD”的可抛出对象。在 main 方法中,调用了 raiseException() 方法。在 catch 块中,我们处理异常并使用 getCause() 方法打印其原因。
package com.tutorialspoint; public class ThrowableDemo { public static void main(String[] args) throws Throwable { try { raiseException(); } catch(Throwable e) { System.out.println(e.getCause()); } } public static void raiseException() throws Throwable { Throwable t = new Throwable("This is new Exception..."); t.initCause(new Throwable("ABCD")); throw t; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
java.lang.Throwable: ABCD
示例:获取 RuntimeException 的初始原因
以下示例演示了 java.lang.Throwable.getCause() 方法的使用。在此程序中,我们定义了一个名为 raiseException() 的方法,在其中定义了一个 RuntimeException,并使用 initCause() 方法将其初始原因设置为带有消息“ABCD”的可抛出对象。在 main 方法中,调用了 raiseException() 方法。在 catch 块中,我们处理异常并使用 getCause() 方法打印其原因。
package com.tutorialspoint; public class ThrowableDemo { public static void main(String[] args) throws Throwable { try { raiseException(); } catch(Exception e) { System.out.println(e.getCause()); } } public static void raiseException() throws RuntimeException { RuntimeException t = new RuntimeException("This is new Exception..."); t.initCause(new Throwable("ABCD")); throw t; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
java.lang.Throwable: ABCD
示例:获取 Exception 的初始原因
以下示例演示了 java.lang.Throwable.getCause() 方法的使用。在此程序中,我们定义了一个名为 raiseException() 的方法,在其中定义了一个 Exception,并使用 initCause() 方法将其初始原因设置为带有消息“ABCD”的可抛出对象。在 main 方法中,调用了 raiseException() 方法。在 catch 块中,我们处理异常并使用 getCause() 方法打印其原因。
package com.tutorialspoint; public class ThrowableDemo { public static void main(String[] args) throws Throwable { try { raiseException(); } catch(Exception e) { System.out.println(e.getCause()); } } public static void raiseException() throws Exception { Exception t = new Exception("This is new Exception..."); t.initCause(new Throwable("ABCD")); throw t; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
java.lang.Throwable: ABCD