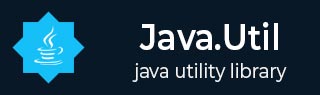
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Arrays binarySearch() 方法
描述
Java Arrays binarySearch(T[] a, T key, Comparator<? super T> c) 方法使用二分查找算法在指定的 Object 数组中搜索指定的值。在调用此方法之前,必须根据指定的 Comparator 对数组进行排序。如果未排序,则结果未定义。
声明
以下是 java.util.Arrays.binarySearch(T[] a, T key, Comparator<? super T> c) 方法的声明
public static <T> int binarySearch(T[] a, T key, Comparator<? super T> c)
参数
a − 要搜索的数组。
key − 要搜索的值。
c − 数组排序使用的比较器。空值表示应使用元素的自然顺序。
返回值
如果搜索键包含在数组中,则此方法返回搜索键的索引;否则,返回 (-(插入点) - 1)。插入点是键将被插入到数组中的位置:大于键的第一个元素的索引,如果数组中的所有元素都小于指定的键,则为 a.length。
异常
ClassCastException − 如果数组包含使用指定的比较器不能相互比较的元素,或者使用此比较器搜索键不能与数组的元素进行比较。
Java Arrays binarySearch(T[] a, int fromIndex, int toIndex, T key, Comparator<? super T> c) 方法
描述
Java Arrays binarySearch(T[] a, int fromIndex, int toIndex, T key, Comparator<? super T> c) 方法使用二分查找算法在一个指定的 Object 数组的范围内搜索指定的值。在调用此方法之前,该范围必须根据指定的 Comparator 进行排序。如果未排序,则结果未定义。
声明
以下是 java.util.Arrays.binarySearch(T[] a, int fromIndex, int toIndex, T key, Comparator<? super T> c) 方法的声明
public static <T> int binarySearch(T[] a, int fromIndex, int toIndex, T key, Comparator<? super T> c)
参数
a − 要搜索的数组。
fromIndex − 要搜索的第一个元素的索引(包含)。
toIndex − 要搜索的最后一个元素的索引(不包含)。
key − 要搜索的值。
c − 数组排序使用的比较器。空值表示应使用元素的自然顺序。
返回值
此方法返回搜索键的索引,如果它包含在数组中,否则返回 (-(插入点) - 1)。插入点是键将被插入到数组中的位置;该范围内大于键的第一个元素的索引,如果该范围内的所有元素都小于指定的键,则为 toIndex。
异常
ClassCastException − 如果搜索键与数组的元素不可比较。
IllegalArgumentException − 如果 fromIndex > toIndex
ArrayIndexOutOfBoundsException − 如果 fromIndex < 0 或 toIndex > a.length
ClassCastException − 如果数组包含使用指定的比较器不能相互比较的元素,或者使用此比较器搜索键不能与数组的元素进行比较。
在对象数组上执行二分查找示例
以下示例演示了 Java Arrays binarySearch(T[], key, comparator) 方法的用法。首先,我们创建了一个 Student 对象数组,对其进行排序并打印。然后对一个值执行二分查找并打印结果。
package com.tutorialspoint; import java.util.Arrays; import java.util.Comparator; public class ArrayDemo { public static void main(String[] args) { // initializing unsorted Object array Student studentsArr[] = new Student[]{new Student(1, "Julie"), new Student(3, "Adam"), new Student(2, "Robert")}; RollNoComparator comparator = new RollNoComparator(); // sorting array Arrays.sort(studentsArr, comparator); // let us print all the elements available in list System.out.println("The sorted Object array is:"); for (Student student : studentsArr) { System.out.println("Student = " + student); } // entering the student to be searched Student searchVal = new Student(1, "Julie"); int retVal = Arrays.binarySearch(studentsArr,searchVal,comparator); System.out.println("The index of student Julie is : " + retVal); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } } class RollNoComparator implements Comparator<Student>{ @Override public int compare(Student o1, Student o2) { return o1.getRollNo()-o2.getRollNo(); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
The sorted Object array is: Student = [ 1, Julie ] Student = [ 2, Robert ] Student = [ 3, Adam ] The index of student Julie is : 0
在对象子数组上执行二分查找示例
以下示例演示了 Java Arrays binarySearch(T[], fromIndex, toIndex, key, comparator) 方法的用法。首先,我们创建了一个 Student 对象数组,对其进行排序并打印。然后对子数组上的一个值执行二分查找并打印结果。
package com.tutorialspoint; import java.util.Arrays; import java.util.Comparator; public class ArrayDemo { public static void main(String[] args) { // initializing unsorted Object array Student studentsArr[] = new Student[]{new Student(1, "Julie"), new Student(3, "Adam"), new Student(2, "Robert")}; RollNoComparator comparator = new RollNoComparator(); // sorting array Arrays.sort(studentsArr, comparator); // let us print all the elements available in list System.out.println("The sorted Object array is:"); for (Student student : studentsArr) { System.out.println("Student = " + student); } // entering the student to be searched Student searchVal = new Student(1, "Julie"); int retVal = Arrays.binarySearch(studentsArr,0,1,searchVal,comparator); System.out.println("The index of student Julie is : " + retVal); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } } class RollNoComparator implements Comparator<Student>{ @Override public int compare(Student o1, Student o2) { return o1.getRollNo()-o2.getRollNo(); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
The sorted Object array is: Student = [ 1, Julie ] Student = [ 2, Robert ] Student = [ 3, Adam ] The index of student Julie is : 0
在对象数组上对不存在的对象执行二分查找示例
以下示例演示了 Java Arrays binarySearch(Object[], key, comparator) 方法的用法。首先,我们创建了一个 Student 对象数组,对其进行排序并打印。然后对数组中不存在的值执行二分查找并打印结果。
package com.tutorialspoint; import java.util.Arrays; import java.util.Comparator; public class ArrayDemo { public static void main(String[] args) { // initializing unsorted Object array Student studentsArr[] = new Student[]{new Student(1, "Julie"), new Student(3, "Adam"), new Student(2, "Robert")}; RollNoComparator comparator = new RollNoComparator(); // sorting array Arrays.sort(studentsArr, comparator); // let us print all the elements available in list System.out.println("The sorted Object array is:"); for (Student student : studentsArr) { System.out.println("Student = " + student); } // entering the student to be searched Student searchVal = new Student(4, "Jene"); int retVal = Arrays.binarySearch(studentsArr,searchVal,comparator); System.out.println("The index of student Jene is : " + retVal); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } } class RollNoComparator implements Comparator<Student>{ @Override public int compare(Student o1, Student o2) { return o1.getRollNo()-o2.getRollNo(); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
The sorted Student array is: Student = [ 1, Julie ] Student = [ 2, Robert ] Student = [ 3, Adam ] The index of student Jene is : -4