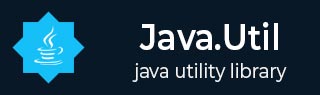
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包附加内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Arrays equals(short[], short[]) 方法
描述
Java Arrays equals(short[] a, short[] a2) 方法返回 true,如果两个指定的短整型数组彼此相等。如果两个数组包含相同顺序的相同元素,则它们相等。如果两个数组引用都为 null,则它们被认为相等。
声明
以下是 java.util.Arrays.equals() 方法的声明
public static boolean equals(short[] a, short[] a2)
参数
a − 这是要测试相等性的数组。
a2 − 这是要测试相等性的另一个数组。
返回值
如果两个数组相等,则此方法返回 true,否则返回 false。
异常
无
Java Arrays equals(short[] a, int aFromIndex, int aToIndex, short[] b, int bFromIndex, int bToIndex) 方法
描述
Java Arrays equals(short[] a, int aFromIndex, int aToIndex, short[] b, int bFromIndex, int bToIndex) 方法返回 true,如果在给定范围内的两个短整型数组彼此相等。如果任何数组为 null,则抛出 NullPointerException。
声明
以下是 java.util.Arrays.compare(short[] a, int aFromIndex, int aToIndex, short[] b, int bFromIndex, int bToIndex) 方法的声明
public static boolean equals(short[] a, int aFromIndex, int aToIndex, short[] b, int bFromIndex, int bToIndex)
参数
a − 这是第一个要测试相等性的数组。
aFromIndex − 这是第一个数组中要测试相等性的第一个元素的索引(包含)。
aToIndex − 这是第一个数组中要测试相等性的最后一个元素的索引(不包含)。
b − 这是第二个要测试相等性的数组。
bFromIndex − 这是第二个数组中要测试相等性的第一个元素的索引(包含)。
bToIndex − 这是第二个数组中要测试相等性的最后一个元素的索引(不包含)。
返回值
如果这两个数组在指定的范围内相等,则此方法返回 true。
异常
IllegalArgumentException − 如果 aFromIndex > aToIndex 或 bFromIndex > bToIndex
ArrayIndexOutOfBoundsException − 如果 aFromIndex < 0 或 aToIndex > a.length 或 bFromIndex < 0 或 bToIndex > b.length
NullPointerException − 如果任一数组为 null
检查短整型数组是否相等的示例
以下示例演示了 Java Arrays equals(short[], short[]) 方法的用法。首先,我们创建了三个短整型数组,并使用 equals(short[], short[]) 方法对它们进行比较。根据结果,打印比较结果。
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initializing three short arrays short[] arr1 = new short[] { 10, 15, 20 }; short[] arr2 = new short[] { 12, 18, 20 }; short[] arr3 = new short[] { 10, 15, 20 }; // comparing arr1 and arr2 boolean retval = Arrays.equals(arr1, arr2); System.out.println("arr1 and arr2 equal: " + retval); // comparing arr1 and arr3 boolean retval2 = Arrays.equals(arr1, arr3); System.out.println("arr1 and arr3 equal: " + retval2); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
arr1 and arr2 equal: false arr1 and arr3 equal: true
检查短整型子数组是否相等的示例
以下示例演示了 Java Arrays equals(short[], int, int, short[], int, int) 方法的用法。首先,我们创建了三个短整型数组,并使用 equals(short[], int, int, short[], int, int) 方法对它们进行比较。根据结果,打印比较结果。
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initializing three short arrays short[] arr1 = new short[] { 10, 15, 20 }; short[] arr2 = new short[] { 12, 18, 20 }; short[] arr3 = new short[] { 10, 15, 20 }; // comparing arr1 and arr2 boolean retval = Arrays.equals(arr1, 0, 2, arr2, 0, 2); System.out.println("arr1 and arr2 equal: " + retval); // comparing arr1 and arr3 boolean retval2 = Arrays.equals(arr1, 0, 2, arr3, 0, 2); System.out.println("arr1 and arr3 equal: " + retval2); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
arr1 and arr2 equal: false arr1 and arr3 equal: true
检查短整型子数组是否相等的示例
以下示例演示了 Java Arrays equals(short[], int, int, short[], int, int) 方法的用法。首先,我们创建了三个短整型数组,并使用 equals(short[], int, int, short[], int, int) 方法比较它们的子数组。根据结果,打印比较结果。
package com.tutorialspoint; import java.util.Arrays; public class ArrayDemo { public static void main(String[] args) { // initializing three short arrays short[] arr1 = new short[] { 10, 15, 20 }; short[] arr2 = new short[] { 12, 18, 20 }; short[] arr3 = new short[] { 10, 15, 20 }; // comparing arr1 and arr2 boolean retval = Arrays.equals(arr1, 2, 3, arr2, 2, 3); System.out.println("Last element of arr1 and arr2 equal: " + retval); // comparing arr1 and arr3 boolean retval2 = Arrays.equals(arr1, 2, 3, arr3, 2, 3); System.out.println("Last element of arr1 and arr3 equal: " + retval2); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Last element of arr1 and arr2 equal: true Last element of arr1 and arr3 equal: true