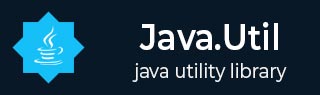
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Arrays spliterator(int[] a) 方法
描述
Java Arrays spliterator(int[] a) 方法返回一个 Spliterator.OfInt,涵盖指定数组的所有元素。
声明
以下是 java.util.Arrays.spliterator(int[] a) 方法的声明
public static Spliterator.OfInt spliterator(int[] array)
参数
a − 这是一个数组,假设在使用过程中不会被修改。
返回值
此方法返回数组元素的 spliterator。
异常
无
Java Arrays spliterator(int[] a, int fromIndex, int toIndex) 方法
描述
Java Arrays spliterator(int[] a, int fromIndex, int toIndex) 方法返回一个 Spliterator.OfInt,涵盖指定数组的指定范围。
声明
以下是 java.util.Arrays.spliterator(int[] a, int fromIndex, int toIndex) 方法的声明
public static Spliterator.OfInt spliterator(int[] array, int fromIndex, int toIndex)
参数
a − 这是一个数组,假设在使用过程中不会被修改。
fromIndex − 这是第一个元素(包含)的索引,将被涵盖。
toIndex − 这是最后一个元素(不包含)的索引,将被涵盖。
返回值
此方法返回数组元素的 spliterator。
异常
ArrayIndexOutOfBoundsException − 如果 fromIndex < 0 或 toIndex > array.length
获取整数数组的 Spliterator 示例
以下示例演示了 Java Arrays spliterator(int[]) 方法的使用。首先,我们创建了一个整数数组。然后使用 spliterator() 方法打印数组。
package com.tutorialspoint; import java.util.Arrays; import java.util.Spliterator; public class ArrayDemo { public static void main(String[] args) { // initialize array int arr[] = { 11, 54, 23, 32, 15, 24, 31, 12 }; System.out.print("Array: [ "); // get the spliterator Spliterator<Integer> spliterator = Arrays.spliterator(arr); // use the spliterator to print each item spliterator.forEachRemaining(i -> System.out.print(i + " ")); System.out.print("]"); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Array: [ 11 54 23 32 15 24 31 12 ]
使用范围获取整数数组的 Spliterator 示例
以下示例演示了 Java Arrays spliterator(int[], int, int) 方法的使用。首先,我们创建了一个整数数组。然后使用 spliterator() 方法打印数组。
package com.tutorialspoint; import java.util.Arrays; import java.util.Spliterator; public class ArrayDemo { public static void main(String[] args) { // initialize array int arr[] = { 11, 54, 23, 32, 15, 24, 31, 12 }; System.out.print("Array: [ "); // get the spliterator Spliterator<Integer> spliterator = Arrays.spliterator(arr, 0, arr.length); // use the spliterator to print each item spliterator.forEachRemaining(i -> System.out.print(i + " ")); System.out.print("]"); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Array: [ 11 54 23 32 15 24 31 12 ]
获取整数子数组的 Spliterator 示例
以下示例演示了 Java Arrays spliterator(int[], int, int) 方法的使用。首先,我们创建了一个整数数组。然后使用 spliterator() 方法打印一个子数组。
package com.tutorialspoint; import java.util.Arrays; import java.util.Spliterator; public class ArrayDemo { public static void main(String[] args) { // initialize array int arr[] = { 11, 54, 23, 32, 15, 24, 31, 12 }; System.out.print("Array: [ "); // get the spliterator Spliterator<Integer> spliterator = Arrays.spliterator(arr, 0, 5); // use the spliterator to print each item spliterator.forEachRemaining(i -> System.out.print(i + " ")); System.out.print("]"); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Array: [ 11 54 23 32 15 ]