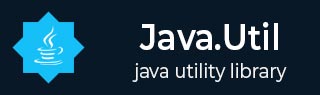
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java 集合 checkedNavigableMap() 方法
描述
checkedNavigableMap(NavigableMap<K, V>, Class<K>, Class<V>) 方法用于获取指定映射的动态类型安全视图。
声明
以下是java.util.Collections.checkedNavigableMap() 方法的声明。
public static <K,V> NavigableMap<K,V> checkedNavigableMap(NavigableMap<K,V> m,Class<K> keyType,Class<V> valueType)
参数
m − 这是要返回动态类型安全视图的可导航映射。
keyType − 这是 m 允许保存的键的类型。
valueType − 这是 m 允许保存的值的类型。
返回值
方法调用返回指定可导航映射的动态类型安全视图。
异常
无
从字符串、整数对映射获取类型安全可导航映射示例
以下示例演示了 Java Collection checkedNavigableMap(NavigableMap,Class,Class) 方法的使用,以获取字符串和整数映射的类型安全视图。我们创建了一个包含一些字符串和整数的 Map 对象。使用 checkedNavigableMap(NavigableMap, String, Integer) 方法,我们获得了字符串和整数的映射,然后将其打印出来。
package com.tutorialspoint; import java.util.Collections; import java.util.NavigableMap; import java.util.TreeMap; public class CollectionsDemo { public static void main(String args[]) { // create map NavigableMap<String,Integer> hmap = new TreeMap<>(); // populate the map hmap.put("1", 1); hmap.put("2", 2); hmap.put("3", 3); hmap.put("4", 4); // get typesafe view of the map NavigableMap<String,Integer> tsmap; tsmap = Collections.checkedNavigableMap(hmap,String.class,Integer.class); System.out.println("Dynamically typesafe view of the map: "+tsmap); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Dynamically typesafe view of the map: {1=1, 2=2, 3=3, 4=4}
从字符串、字符串对映射获取类型安全可导航映射示例
以下示例演示了 Java Collection checkedNavigableMap(NavigableMap,Class,Class) 方法的使用,以获取字符串和字符串映射的类型安全视图。我们创建了一个包含一些字符串和字符串的 Map 对象。使用 checkedNavigableMap(NavigableMap, String, String) 方法,我们获得了字符串和字符串的映射,然后将其打印出来。
package com.tutorialspoint; import java.util.Collections; import java.util.NavigableMap; import java.util.TreeMap; public class CollectionsDemo { public static void main(String args[]) { // create map NavigableMap<String,String> hmap = new TreeMap<>(); // populate the map hmap.put("1", "A"); hmap.put("2", "B"); hmap.put("3", "C"); hmap.put("4", "D"); // get typesafe view of the map NavigableMap<String,String> tsmap; tsmap = Collections.checkedNavigableMap(hmap,String.class,String.class); System.out.println("Dynamically typesafe view of the map: "+tsmap); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Dynamically typesafe view of the map: {1=A, 2=B, 3=C, 4=D}
从字符串、对象对映射获取类型安全可导航映射示例
以下示例演示了 Java Collection checkedCollection(NavigableMap,Class) 方法的使用,以获取 Student 对象映射的类型安全视图。我们创建了一个包含一些 Student 对象的映射,并打印了原始映射。使用 checkedCollection(NavigableMap, Student) 方法,我们获得了字符串的可导航映射,然后将其打印出来。
package com.tutorialspoint; import java.util.Collections; import java.util.NavigableMap; import java.util.TreeMap; public class CollectionsDemo { public static void main(String[] args) { // create map NavigableMap<String,Student> hmap = new TreeMap<>(); // populate the map hmap.put("1", new Student(1, "Julie")); hmap.put("2", new Student(2, "Robert")); hmap.put("3", new Student(3, "Adam")); hmap.put("4", new Student(4, "Jene")); // get typesafe view of the map NavigableMap<String,Student> tsmap; tsmap = Collections.checkedNavigableMap(hmap,String.class,Student.class); System.out.println("Dynamically typesafe view of the map: "+tsmap); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Dynamically typesafe view of the map: {1=[ 1, Julie ], 2=[ 2, Robert ], 3=[ 3, Adam ], 4=[ 4, Jene ]}