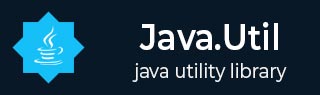
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java 集合 copy() 方法
描述
Java 集合 copy(List<? super T>, List<? extends T>) 方法用于将一个列表中的所有元素复制到另一个列表。
声明
以下是 java.util.Collections.copy() 方法的声明。
public static <T> void copy(List<? super T> dest,List<? extends T> src)
参数
dest − 这是目标列表。
src − 这是源列表。
返回值
无
异常
IndexOutOfBoundsException − 如果目标列表太小而无法容纳整个源列表,则抛出此异常。
UnsupportedOperationException − 如果目标列表的列表迭代器不支持 set 操作,则抛出此异常。
复制整数列表示例
以下示例演示了如何使用 Java 集合 copy(List,List) 方法复制整数列表。我们创建了两个包含一些整数的列表。使用 copy(List,List) 方法,我们将一个列表的内容复制到另一个列表,同时覆盖现有内容。
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<Integer> srclst = new ArrayList<>(); List<Integer> destlst = new ArrayList<>(); // populate two lists srclst.add(1); srclst.add(2); srclst.add(3); destlst.add(4); destlst.add(5); destlst.add(6); destlst.add(7); // copy into dest list Collections.copy(destlst, srclst); System.out.println("Value of source list: "+srclst); System.out.println("Value of destination list: "+destlst); } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Value of source list: [1, 2, 3] Value of destination list: [1, 2, 3, 7]
复制字符串列表示例
以下示例演示了如何使用 Java 集合 copy(List,List) 方法复制字符串列表。我们创建了两个包含一些字符串的列表。使用 copy(List,List) 方法,我们将一个列表的内容复制到另一个列表,同时覆盖现有内容。
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<String> srclst = new ArrayList<>(); List<String> destlst = new ArrayList<>(); // populate two lists srclst.add("A"); srclst.add("B"); srclst.add("C"); destlst.add("D"); destlst.add("E"); destlst.add("F"); destlst.add("G"); // copy into dest list Collections.copy(destlst, srclst); System.out.println("Value of source list: "+srclst); System.out.println("Value of destination list: "+destlst); } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Value of source list: [A, B, C] Value of destination list: [A, B, C, G]
复制对象列表示例
以下示例演示了如何使用 Java 集合 copy(List,List) 方法复制 Student 对象列表。我们创建了两个包含一些 Student 对象的列表。使用 copy(List,List) 方法,我们将一个列表的内容复制到另一个列表,同时覆盖现有内容。
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<Student> srclst = new ArrayList<>(Arrays.asList(new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"))); List<Student> destlst = new ArrayList<>(Arrays.asList(new Student(4, "Jene"), new Student(5, "Julie"), new Student(6, "Trus"))); // copy into dest list Collections.copy(destlst, srclst); System.out.println("Value of source list: "+srclst); System.out.println("Value of destination list: "+destlst); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Value of source list: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]] Value of destination list: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]]
java_util_collections.htm
广告