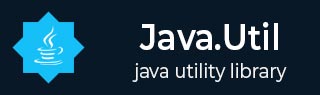
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java 集合 disjoint() 方法
描述
Java 集合 disjoint(Collection<?>, Collection<?>) 方法用于判断两个指定的集合是否没有任何共同元素,如果有则返回 'false',否则返回 'true'。
声明
以下是 java.util.Collections.disjoint() 方法的声明。
public static boolean disjoint(Collection<?> c1,Collection<?> c2)
参数
c1 − 一个集合。
c2 − 另一个集合。
返回值
布尔值,表示两个集合是否不相交。
异常
NullPointerException − 如果任一集合为 null,则抛出此异常。
比较整数列表的公共值示例
以下示例演示了如何使用 Java 集合 disjoint(Collection,Collection) 方法来比较整数列表。我们创建了两个包含一些整数的列表。使用 disjoint(Collection,Collection) 方法,我们将一个列表的内容与另一个列表的内容进行比较,查看是否存在任何公共元素,然后打印结果。
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<Integer> srclst = new ArrayList<>(); List<Integer> destlst = new ArrayList<>(); // populate two lists srclst.add(1); srclst.add(2); srclst.add(3); destlst.add(4); destlst.add(5); destlst.add(6); destlst.add(7); // check elements in both collections boolean iscommon = Collections.disjoint(srclst, destlst); System.out.println("No commom elements: "+iscommon); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
No commom elements: true
比较字符串列表的公共值示例
以下示例演示了如何使用 Java 集合 disjoint(Collection,Collection) 方法来比较字符串列表。我们创建了两个包含一些字符串的列表。使用 disjoint(Collection,Collection) 方法,我们将一个列表的内容与另一个列表的内容进行比较,查看是否存在任何公共元素,然后打印结果。
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<String> srclst = new ArrayList<>(); List<String> destlst = new ArrayList<>(); // populate two lists srclst.add("A"); srclst.add("B"); srclst.add("C"); destlst.add("D"); destlst.add("E"); destlst.add("F"); destlst.add("G"); // check elements in both collections boolean iscommon = Collections.disjoint(srclst, destlst); System.out.println("No commom elements: "+iscommon); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
No commom elements: true
比较对象列表的公共值示例
以下示例演示了如何使用 Java 集合 disjoint(Collection,Collection) 方法来比较学生对象列表。我们创建了两个包含一些学生对象的列表。使用 disjoint(Collection,Collection) 方法,我们将一个列表的内容与另一个列表的内容进行比较,查看是否存在任何公共元素,然后打印结果。
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<Student> srclst = new ArrayList<>(Arrays.asList(new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"))); List<Student> destlst = new ArrayList<>(Arrays.asList(new Student(4, "Jene"), new Student(5, "Julie"), new Student(6, "Trus"))); // check elements in both collections boolean iscommon = Collections.disjoint(srclst, destlst); System.out.println("No commom elements: "+iscommon); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
No commom elements: true