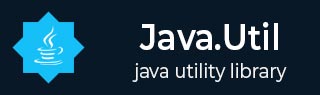
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包扩展
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java 集合 emptyListIterator() 方法
描述
Java 集合 emptyListIterator() 方法用于获取空的列表迭代器。ListIterator 为空,其 hasNext 方法始终返回 false。next() 方法调用会抛出 NoSuchElementException,而 remove() 方法会抛出 IllegalStateException。
声明
以下是 Java 集合 emptyListIterator() 方法的声明。
public static <T> ListIterator<T> emptyListIterator()
参数
无
返回值
无
异常
无
获取整数的空列表迭代器示例
以下示例演示了如何使用 Java 集合 emptyListIterator() 方法获取整数的空列表迭代器。我们使用 emptyListIterator() 方法创建了一个空的列表迭代器,然后检查列表迭代器是否包含元素。
package com.tutorialspoint; import java.util.Collections; import java.util.ListIterator; public class CollectionsDemo { public static void main(String args[]) { // create an empty list ListIterator<Integer> emptyListIterator = Collections.emptyListIterator(); System.out.println("Created empty list iterator, it has elements: "+emptyListIterator.hasNext()); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Created empty list iterator, it has elements: false
获取字符串的空列表迭代器示例
以下示例演示了如何使用 Java 集合 emptyListIterator() 方法获取字符串的空列表迭代器。我们使用 emptyListIterator() 方法创建了一个空的列表迭代器,然后检查列表迭代器是否包含元素。
package com.tutorialspoint; import java.util.Collections; import java.util.ListIterator; public class CollectionsDemo { public static void main(String args[]) { // create an empty list ListIterator<String> emptyListIterator = Collections.emptyListIterator(); System.out.println("Created empty list iterator, it has elements: "+emptyListIterator.hasNext()); } }
输出
让我们编译并运行上述程序,这将产生以下结果。由于列表是不可变的,因此将抛出异常。
Created empty list iterator, it has elements: false
获取对象的空列表迭代器示例
以下示例演示了如何使用 Java 集合 emptyListIterator() 方法获取 Student 对象的空列表迭代器。我们使用 emptyListIterator() 方法创建了一个空的列表迭代器,然后检查列表迭代器是否包含元素。
package com.tutorialspoint; import java.util.Collections; import java.util.ListIterator; public class CollectionsDemo { public static void main(String args[]) { // create an empty list ListIterator<Student> emptyListIterator = Collections.emptyListIterator(); System.out.println("Created empty list iterator, it has elements: "+emptyListIterator.hasNext()); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上述程序,这将产生以下结果。由于列表是不可变的,因此将抛出异常。
Created empty list iterator, it has elements: false
java_util_collections.htm
广告