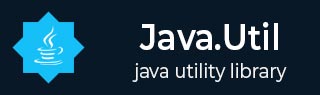
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包扩展
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java 集合 list() 方法
描述
Java Collections list(Enumeration<T>) 方法用于获取一个数组列表,该列表包含指定枚举返回的元素,元素顺序与枚举返回的顺序相同。
声明
以下是 java.util.Collections.list() 方法的声明。
public static <T> ArrayList<T> list(Enumeration<T> e)
参数
e - 这是为返回的数组列表提供元素的枚举。
返回值
方法调用返回指定目标列表在指定源列表中最后一次出现的起始位置,如果不存在这样的出现,则返回 -1。
异常
无
从整数向量获取列表示例
以下示例演示了 Java Collection list(Enumeration) 方法的用法。我们创建了一个向量对象,并用一些整数填充它。然后检索其枚举,并使用 list(Enumeration) 方法准备并打印一个数组列表。
package com.tutorialspoint; import java.util.ArrayList; import java.util.Collections; import java.util.Enumeration; import java.util.List; import java.util.Vector; public class CollectionsDemo { public static void main(String args[]) { // create vector and array list List<Integer> arrlist = new ArrayList<>(); Vector<Integer> v = new Vector<>(); // populate the vector v.add(1); v.add(2); v.add(3); v.add(4); v.add(5); // create enumeration Enumeration<Integer> e = v.elements(); // get the list arrlist = Collections.list(e); System.out.println("Value of returned list: "+arrlist); } }
输出
让我们编译并运行上述程序,这将产生以下结果 -
Value of returned list: [1, 2, 3, 4, 5]
从字符串向量获取列表示例
以下示例演示了 Java Collection list(Enumeration) 方法的用法。我们创建了一个向量对象,并用一些字符串填充它。然后检索其枚举,并使用 list(Enumeration) 方法准备并打印一个数组列表。
package com.tutorialspoint; import java.util.ArrayList; import java.util.Collections; import java.util.Enumeration; import java.util.List; import java.util.Vector; public class CollectionsDemo { public static void main(String args[]) { // create vector and array list List<String> arrlist = new ArrayList<>(); Vector<String> v = new Vector<>(); // populate the vector v.add("A"); v.add("B"); v.add("C"); v.add("D"); v.add("E"); // create enumeration Enumeration<String> e = v.elements(); // get the list arrlist = Collections.list(e); System.out.println("Value of returned list: "+arrlist); } }
输出
让我们编译并运行上述程序,这将产生以下结果 -
Value of returned list: [A, B, C, D, E]
从对象向量获取列表示例
以下示例演示了 Java Collection list(Enumeration) 方法的用法。我们创建了一个向量对象,并用一些 Student 对象填充它。然后检索其枚举,并使用 list(Enumeration) 方法准备并打印一个数组列表。
package com.tutorialspoint; import java.util.ArrayList; import java.util.Collections; import java.util.Enumeration; import java.util.List; import java.util.Vector; public class CollectionsDemo { public static void main(String args[]) { // create vector and array list List<Student> arrlist = new ArrayList<>(); Vector<Student> v = new Vector<>(); // populate the vector v.add(new Student(1, "Julie")); v.add(new Student(2, "Robert")); v.add(new Student(3, "Adam")); v.add(new Student(4, "Jene")); v.add(new Student(5, "Joe")); // create enumeration Enumeration<Student> e = v.elements(); // get the list arrlist = Collections.list(e); System.out.println("Value of returned list: "+arrlist); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
输出
让我们编译并运行上述程序,这将产生以下结果 -
Value of returned list: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ], [ 4, Jene ], [ 5, Joe ]]
java_util_collections.htm
广告