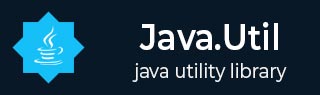
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包其他内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java 集合框架 unmodifiableList() 方法
描述
Java 集合框架 unmodifiableList() 方法用于返回指定列表的不可修改视图。
声明
以下是 java.util.Collections.unmodifiableList() 方法的声明。
public static <T> List<T> unmodifiableList(List<? extends T> list)
参数
list − 这是要返回不可修改视图的列表。
返回值
方法调用返回指定列表的不可修改视图。
异常
无
从可变整数列表获取不可变列表示例
以下示例演示了 Java 集合框架 unmodifiableList(List) 方法的使用。我们创建了一个包含一些整数的 List 对象。使用 unmodifiableList(List) 方法,我们获取了列表的不可变版本并打印了列表。
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Integer> list = new ArrayList<>(Arrays.asList(1,2,3,4,5)); // immutable version of list List<Integer> c = Collections.unmodifiableList(list); System.out.println("Immutable list: "+ c); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Immutable list: [1, 2, 3, 4, 5]
从可变字符串列表获取不可变列表示例
以下示例演示了 Java 集合框架 unmodifiableList(List) 方法的使用。我们创建了一个包含一些字符串的 List 对象。使用 unmodifiableList(List) 方法,我们获取了列表的不可变版本并打印了列表。
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<String> list = new ArrayList<>(Arrays.asList("Welcome","to","Tutorialspoint")); // immutable version of list List<String> c = Collections.unmodifiableList(list); System.out.println("Immutable list: "+ c); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Immutable list: [Welcome, to, Tutorialspoint]
从可变对象列表获取不可变列表示例
以下示例演示了 Java 集合框架 unmodifiableList(List) 方法的使用。我们创建了一个包含一些 Student 对象的 List 对象。使用 unmodifiableList(List) 方法,我们获取了列表的不可变版本并打印了列表。
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Student> list = new ArrayList<>(Arrays.asList(new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"))); // immutable version of list List<Student> c = Collections.unmodifiableList(list); System.out.println("Immutable list: "+ c); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Immutable list: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]]
java_util_collections.htm
广告