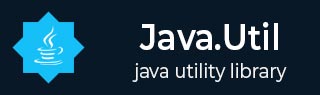
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包补充
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Dictionary size() 方法
描述
Java Dictionary size() 方法返回此字典中的条目数(不同的键)。
声明
以下是 java.util.Dictionary.size() 方法的声明
public abstract int size()
参数
无
返回值
此方法返回此字典中的键数。
异常
无
获取整数、整数对字典的大小示例
以下示例演示了 Java Dictionary size() 方法的用法。我们使用 Integer, Integer 的 Hashtable 对象创建一个字典实例。我们使用 size() 方法检查字典的状态并打印结果。然后我们向其中添加了一些元素,并使用 size() 方法检查字典的状态并打印结果。
package com.tutorialspoint; import java.util.Dictionary; import java.util.Hashtable; public class DictionaryDemo { public static void main(String[] args) { // create a new hashtable Dictionary<Integer, Integer> dictionary = new Hashtable<>(); System.out.println(dictionary.size()); // add 2 elements dictionary.put(1, 1); dictionary.put(2, 2); System.out.println(dictionary.size()); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
0 2
获取整数、字符串对字典的大小示例
以下示例演示了 Java Dictionary size() 方法的用法。我们使用 Integer, String 对的 Hashtable 对象创建一个字典实例。我们使用 size() 方法检查字典的状态并打印结果。然后我们向其中添加了一些元素,并使用 size() 方法检查字典的状态并打印结果。
package com.tutorialspoint; import java.util.Dictionary; import java.util.Hashtable; public class DictionaryDemo { public static void main(String[] args) { // create a new hashtable Dictionary<Integer, String> dictionary = new Hashtable<>(); System.out.println(dictionary.size()); // add 2 elements dictionary.put(1, "One"); dictionary.put(2, "Two"); System.out.println(dictionary.size()); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
0 2
获取整数、对象对字典的大小示例
以下示例演示了 Java Dictionary size() 方法的用法。我们使用 Integer, Student 对的 Hashtable 对象创建一个字典实例。我们使用 size() 方法检查字典的状态并打印结果。然后我们向其中添加了一些元素,并使用 size() 方法检查字典的状态并打印结果。
package com.tutorialspoint; import java.util.Dictionary; import java.util.Hashtable; public class DictionaryDemo { public static void main(String[] args) { // create a new hashtable Dictionary<Integer, Student> dictionary = new Hashtable<>(); System.out.println(dictionary.size()); // add 2 elements dictionary.put(1, new Student(1, "Julie")); dictionary.put(2, new Student(2, "Robert")); System.out.println(dictionary.size()); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
0 2
java_util_dictionary.htm
广告