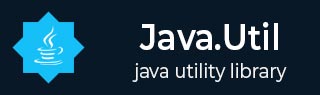
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java HashMap size() 方法
描述
Java HashMap size() 方法用于返回此映射中的键值映射数。
声明
以下是 java.util.HashMap.size() 方法的声明。
public int size()
参数
无
返回值
方法调用返回此映射中的键值映射数。
异常
无
获取整数、整数对 HashMap 大小的示例
以下示例演示了如何使用 Java HashMap size() 方法获取 Map 的大小。我们创建了一个 Integer,Integer 对的 Map 对象。然后添加了一些条目,打印了 map。使用 size() 方法检索并打印 map 的大小。
package com.tutorialspoint; import java.util.HashMap; public class HashMapDemo { public static void main(String args[]) { // create hash map HashMap<Integer,Integer> newmap = new HashMap<>(); // populate hash map newmap.put(1, 1); newmap.put(2, 2); newmap.put(3, 3); System.out.println("Initial map elements: " + newmap); System.out.println("Size of the map: " + newmap.size()); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Initial map elements: {1=1, 2=2, 3=3} Size of the map: 3
获取整数、字符串对 HashMap 大小的示例
以下示例演示了如何使用 Java HashMap size() 方法获取 Map 的大小。我们创建了一个 Integer,String 对的 Map 对象。然后添加了一些条目,打印了 map。使用 size() 方法检索并打印 map 的大小。
package com.tutorialspoint; import java.util.HashMap; public class HashMapDemo { public static void main(String args[]) { // create hash map HashMap<Integer,String> newmap = new HashMap<>(); // populate hash map newmap.put(1, "tutorials"); newmap.put(2, "point"); newmap.put(3, "is best"); System.out.println("Initial map elements: " + newmap); System.out.println("Size of the map: " + newmap.size()); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Initial map elements: {1=tutorials, 2=point, 3=is best} Size of the map: 3
获取整数、学生对 HashMap 大小的示例
以下示例演示了如何使用 Java HashMap size() 方法获取 Map 的大小。我们创建了一个 Integer,Student 对的 Map 对象。然后添加了一些条目,打印了 map。使用 size() 方法检索并打印 map 的大小。
package com.tutorialspoint; import java.util.HashMap; public class HashMapDemo { public static void main(String args[]) { // create hash map HashMap<Integer,Student> newmap = new HashMap<>(); // populate hash map newmap.put(1, new Student(1, "Julie")); newmap.put(2, new Student(2, "Robert")); newmap.put(3, new Student(3, "Adam")); System.out.println("Initial map elements: " + newmap); System.out.println("Size of the map: " + newmap.size()); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Initial map elements: {1=[ 1, Julie ], 2=[ 2, Robert ], 3=[ 3, Adam ]} Size of the map: 3
java_util_hashmap.htm
广告