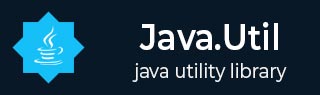
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java HashSet clone() 方法
描述
Java HashSet clone() 方法用于返回此 HashSet 实例的浅拷贝。
声明
以下是 java.util.HashSet.clone() 方法的声明。
public Object clone()
参数
无
返回值
方法调用返回此集合的浅拷贝。
异常
无
克隆整数 HashSet 示例
以下示例演示了如何使用 Java HashSet clone() 方法创建 HashSet 的浅拷贝。我们创建了两个整数 HashSet 对象。然后使用 add() 方法向一个集合中添加一些条目,然后打印该集合。现在使用 clone() 方法克隆集合并打印。
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Integer> newset = new HashSet <>(); HashSet <Integer> newset1 = new HashSet <>(); // populate hash set newset.add(1); newset.add(2); newset.add(3); // checking elements in hash set System.out.println("Hash set values: "+ newset); // clone the set newset1 = (HashSet<Integer>) newset.clone(); // print the set System.out.println("Cloned Hash set values: "+ newset1); } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Hash set values: [1, 2, 3] Cloned Hash set values: [1, 2, 3]
克隆字符串 HashSet 示例
以下示例演示了如何使用 Java HashSet clone() 方法创建 HashSet 的浅拷贝。我们创建了两个字符串 HashSet 对象。然后使用 add() 方法向一个集合中添加一些条目,然后打印该集合。现在使用 clone() 方法克隆集合并打印。
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <String> newset = new HashSet <>(); HashSet <String> newset1 = new HashSet <>(); // populate hash set newset.add("Learning"); newset.add("Easy"); newset.add("Simply"); // checking elements in hash set System.out.println("Hash set values: "+ newset); // clone the set newset1 = (HashSet<String>) newset.clone(); // print the set System.out.println("Cloned Hash set values: "+ newset1); } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Hash set values: [Learning, Easy, Simply] Cloned Hash set values: [Learning, Easy, Simply]
克隆对象 HashSet 示例
以下示例演示了如何使用 Java HashSet clone() 方法创建 HashSet 的浅拷贝。我们创建了两个 Student 对象的 HashSet 对象。然后使用 add() 方法向一个集合中添加一些条目,然后打印该集合。现在使用 clone() 方法克隆集合并打印。
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Student> newset = new HashSet <>(); HashSet <Student> newset1 = new HashSet <>(); // populate hash set newset.add(new Student(1, "Julie")); newset.add(new Student(2, "Robert")); newset.add(new Student(3, "Adam")); // checking elements in hash set System.out.println("Hash set values: "+ newset); // clone the set newset1 = (HashSet<Student>) newset.clone(); // print the set System.out.println("Cloned Hash set values: "+ newset1); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上面的程序,这将产生以下结果。
Hash set values: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]] Cloned Hash set values: [[ 2, Robert ], [ 1, Julie ], [ 3, Adam ]]
java_util_hashset.htm
广告