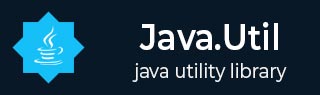
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java HashSet remove() 方法
描述
Java HashSet remove(Object o) 方法用于从该集合中移除指定的元素(如果存在)。
声明
以下是 java.util.HashSet.remove() 方法的声明。
public boolean remove(Object o)
参数
o − 这是要从该集合中移除的对象(如果存在)。
返回值
如果集合包含指定的元素,则方法调用返回“true”。
异常
无
从整数 HashSet 中移除条目示例
以下示例演示了 Java HashSet remove() 方法的使用,用于从 HashSet 中移除条目。我们创建了一个 Integer 类型的 HashSet 对象。然后使用 add() 方法添加了一些条目,并打印该集合。现在,使用 remove() 方法移除一个条目并再次打印该集合。
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Integer> newset = new HashSet <>(); // populate hash set newset.add(1); newset.add(2); newset.add(3); // checking elements in hash set System.out.println("Hash set values: "+ newset); // remove 2 from the set newset.remove(2); // print the set System.out.println("Hash set values after remove operation: "+ newset); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Hash set values: [1, 2, 3] Hash set values after remove operation: [1, 3]
从字符串 HashSet 中移除条目示例
以下示例演示了 Java HashSet remove() 方法的使用,用于从 HashSet 中移除条目。我们创建了一个 String 类型的 HashSet 对象。然后使用 add() 方法添加了一些条目,并打印该集合。现在,使用 remove() 方法移除一个条目并再次打印该集合。
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <String> newset = new HashSet <>(); // populate hash set newset.add("Learning"); newset.add("Easy"); newset.add("Simply"); // checking elements in hash set System.out.println("Hash set values: "+ newset); // remove "Easy" from the set newset.remove("Easy"); // print the set System.out.println("Hash set values after remove operation: "+ newset); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Hash set values: [Learning, Easy, Simply] Hash set values after remove operation: [Learning, Simply]
从对象 HashSet 中移除条目示例
以下示例演示了 Java HashSet remove() 方法的使用,用于从 HashSet 中移除条目。我们创建了一个 Student 对象类型的 HashSet 对象。然后使用 add() 方法添加了一些条目,并打印该集合。现在,使用 remove() 方法移除一个条目并再次打印该集合。
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Student> newset = new HashSet <>(); // populate hash set newset.add(new Student(1, "Julie")); newset.add(new Student(2, "Robert")); newset.add(new Student(3, "Adam")); // checking elements in hash set System.out.println("Hash set values: "+ newset); // remove Robert from the set newset.remove(new Student(2, "Robert")); // print the set System.out.println("Hash set values after remove operation: "+ newset); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { if(obj == null) return false; Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } @Override public int hashCode() { return rollNo + name.hashCode(); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
Hash set values: [[ 2, Robert ], [ 1, Julie ], [ 3, Adam ]] Hash set values after remove operation: [[ 1, Julie ], [ 3, Adam ]]
java_util_hashset.htm
广告