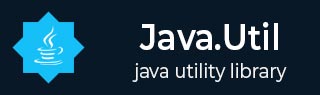
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包扩展
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java LinkedList contains() 方法
描述
Java LinkedList contains(Object) 方法用于判断此列表是否包含指定元素。为了使此操作成功,该对象应该已实现 equals() 方法。
声明
以下是 java.util.LinkedList.contains() 方法的声明
public boolean contains(Object o)
参数
o − 要在此列表中测试其是否存在元素。
返回值
如果此列表包含指定的元素,则此方法返回 true。
异常
无
在整数 LinkedList 中检查元素是否存在示例
以下示例演示了 Java LinkedList contains() 方法的使用。我们使用的是整数。我们将添加一些元素,然后检查特定元素是否存在。
package com.tutorialspoint; import java.util.LinkedList; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<Integer> linkedList = new LinkedList<>(); // use add() method to add elements in the linkedList linkedList.add(20); linkedList.add(30); linkedList.add(10); linkedList.add(18); // let us print all the elements available in linkedList System.out.println("LinkedList = " + linkedList); // linkedList contains element 10 if (linkedList.contains(10)) { System.out.println("element 10 is present in the linkedList"); } else { System.out.println("element 10 is not present in the linkedList"); } // linkedList does not contain element 25 if (linkedList.contains(25)) { System.out.println("element 25 is present in the linkedList"); } else { System.out.println("element 25 is not present in the linkedList"); } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
LinkedList = [20, 30, 10, 18] element 10 is present in the linkedList element 25 is not present in the linkedList
在字符串 LinkedList 中检查元素是否存在示例
以下示例演示了 Java LinkedList contains() 方法与字符串一起使用。我们将添加一些元素,然后检查特定元素是否存在。
package com.tutorialspoint; import java.util.LinkedList; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<String> linkedList = new LinkedList<>(); // use add() method to add elements in the linkedList linkedList.add("Welcome"); linkedList.add("to"); linkedList.add("tutorialspoint"); linkedList.add(".com"); // let us print all the elements available in linkedList System.out.println("LinkedList = " + linkedList); // linkedList contains element tutorialspoint if (linkedList.contains("tutorialspoint")) { System.out.println("element tutorialspoint is present in the linkedList"); } else { System.out.println("element tutorialspoint is not present in the linkedList"); } // linkedList does not contain element html if (linkedList.contains("html")) { System.out.println("element html is present in the linkedList"); } else { System.out.println("element html is not present in the linkedList"); } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
LinkedList = [Welcome, to, tutorialspoint, .com] element tutorialspoint is present in the deque element html is not present in the deque
在对象 LinkedList 中检查元素是否存在示例
以下示例演示了 Java LinkedList contains() 方法与 Student 对象一起使用。我们将添加一些元素,然后检查特定元素是否存在。
package com.tutorialspoint; import java.util.LinkedList; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<Student> linkedList = new LinkedList<>(); // use add() method to add elements in the linkedList linkedList.add(new Student(1, "Julie")); linkedList.add(new Student(2, "Robert")); linkedList.add(new Student(3, "Adam")); // let us print all the elements available in linkedList System.out.println("LinkedList = " + linkedList); // linkedList contains element Robert if (linkedList.contains(new Student(2, "Robert"))) { System.out.println("Student Robert is present in the linkedList"); } else { System.out.println("Student Robert is not present in the linkedList"); } // linkedList does not contain element Jane if (linkedList.contains(new Student(4, "Jane"))) { System.out.println("Student Jane is present in the linkedList"); } else { System.out.println("Student Jane is not present in the linkedList"); } } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
输出
让我们编译并运行以上程序,这将产生以下结果:
LinkedList = [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]] Student Robert is present in the deque Student Jane is not present in the deque
java_util_linkedlist.htm
广告