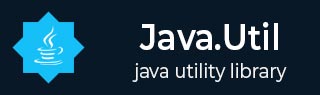
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包补充说明
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java LinkedList push() 方法
描述
Java LinkedList push(E e) 方法将元素 E 推入由该 linkedList 表示的栈中。本质上是将元素添加到 LinkedList 对象的头部。
声明
以下是 java.util.LinkedList.push() 方法的声明
public void push(E e)
参数
e − 要推入 linkedList 的元素。
返回值
此方法不返回任何值。
异常
NullPointerException − 如果指定的元素为 null。
将元素添加到整数 LinkedList 头部的示例
以下示例演示了 Java LinkedList push(E) 方法的用法。在此示例中,我们使用整数。首先,我们将使用 add() 方法向 linkedList 添加一些项目,然后使用 push() 方法将元素添加到栈中。然后,我们再次使用 add() 方法添加更多元素,并打印 arraydeque 以检查 linkedList 中的插入是否按我们期望的顺序进行。
package com.tutorialspoint; import java.util.LinkedList; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<Integer> linkedList = new LinkedList<>(); // use add() method to add elements in the linkedList linkedList.add(4); linkedList.add(5); linkedList.add(6); // use push() method to add element at the front of the linkedList linkedList.push(3); linkedList.push(2); linkedList.push(1);//now, element 1 will be at the front // these elements will be added in continuation with linkedList.add(6) linkedList.add(7); linkedList.add(8); // let us print all the elements available in linkedList System.out.println("LinkedList = " + linkedList); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
LinkedList = [1, 2, 3, 4, 5, 6, 7, 8]
将元素添加到字符串 LinkedList 头部的示例
以下示例演示了 Java LinkedList push(E) 方法的用法。在此示例中,我们使用字符串。首先,我们将使用 add() 方法向 linkedList 添加一些项目,然后使用 push() 方法将元素添加到栈中。然后,我们再次使用 add() 方法添加更多元素,并打印 arraydeque 以检查 linkedList 中的插入是否按我们期望的顺序进行。
package com.tutorialspoint; import java.util.LinkedList; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<String> linkedList = new LinkedList<>(); // use add() method to add elements in the linkedList linkedList.add("D"); linkedList.add("E"); linkedList.add("F"); // use push() method to add element to the stack linkedList.push("C"); linkedList.push("B"); linkedList.push("A");//now, element A will be at the front // these elements will be added in continuation with linkedList.add("F") linkedList.add("G"); linkedList.add("H"); // let us print all the elements available in linkedList System.out.println("LinkedList = " + linkedList); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
LinkedList = [A, B, C, D, E, F, G, H]
将元素添加到对象 LinkedList 头部的示例
以下示例演示了 Java LinkedList push(E) 方法的用法。在此示例中,我们使用 Student 对象。首先,我们将使用 add() 方法向 linkedList 添加一些项目,然后使用 push() 方法将元素添加到 linkedList 的头部。然后,我们再次使用 add() 方法添加更多元素,并打印 arraydeque 以检查 linkedList 中的插入是否按我们期望的顺序进行。
package com.tutorialspoint; import java.util.LinkedList; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<Student> linkedList = new LinkedList<>(); // use add() method to add elements in the linkedList linkedList.add(new Student(4, "Julie")); linkedList.add(new Student(5, "Robert")); linkedList.add(new Student(6, "Adam")); // use push() method to add element to the stack linkedList.push(new Student(3, "Rohan")); linkedList.push(new Student(2, "Sohan")); linkedList.push(new Student(1, "Mohan"));//now, Student 1 will be at the front // these elements will be added in continuation with linkedList.add(new Student(6, "Adam")) linkedList.add(new Student(7, "Ali")); linkedList.add(new Student(8, "Ahmad")); // let us print all the elements available in linkedList System.out.println("LinkedList = " + linkedList); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上述程序,这将产生以下结果:
LinkedList = [[ 1, Mohan ], [ 2, Sohan ], [ 3, Rohan ], [ 4, Julie ], [ 5, Robert ], [ 6, Adam ], [ 7, Ali ], [ 8, Ahmad ]]