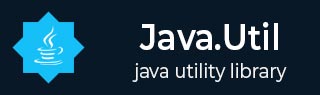
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包补充
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java ListResourceBundle handleKeySet() 方法
描述
Java ListResourceBundle handleKeySet() 方法返回仅包含在此 ResourceBundle 中的键的集合。
声明
以下是 java.util.ListResourceBundle.handleKeySet() 方法的声明
protected Set<String> handleKeySet()
参数
无
返回值
此方法返回仅包含在此 ResourceBundle 中的键的集合。
异常
无
获取字符串、整数对 ListResourceBundle 的键集示例
以下示例演示了 Java ListResourceBundle handleKeySet() 方法的使用,用于迭代 ListResourceBundle 的键。我们创建了一个 ListResourceBundle 对象,其内容为字符串、整数对的二维数组。然后通过重写 getContents() 方法添加一些条目,然后在重写的 handleKeySet() 方法中检索键的枚举并进行迭代以获取键。在主方法中,我们使用 handleKeySet() 方法获取键的集合。
package com.tutorialspoint; import java.util.Enumeration; import java.util.HashSet; import java.util.ListResourceBundle; import java.util.Set; // create a class that extends to ListResourceBundle class MyResources extends ListResourceBundle { // get contents must be implemented protected Object[][] getContents() { return new Object[][] { {"1", 1}, {"2", 2}, {"3", 3} }; } protected Set<String> handleKeySet() { Set<String> set = new HashSet<String>(); // get the keys Enumeration<String> enumeration = getKeys(); // print the keys while (enumeration.hasMoreElements()) { set.add(enumeration.nextElement()); } return set; } } public class ListResourceBundleDemo { public static void main(String[] args) { // create a new MyResources instance MyResources mr = new MyResources(); // get the set of Keys Set<String> keys = mr.handleKeySet(); // print each key keys.forEach(System.out::println); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
1 2 3
获取字符串、字符串对 ListResourceBundle 的键集示例
以下示例演示了 Java ListResourceBundle handleKeySet() 方法的使用,用于迭代 ListResourceBundle 的键。我们创建了一个 ListResourceBundle 对象,其内容为字符串、字符串对的二维数组。然后通过重写 getContents() 方法添加一些条目,然后在重写的 handleKeySet() 方法中检索键的枚举并进行迭代以获取键。在主方法中,我们使用 handleKeySet() 方法获取键的集合。
package com.tutorialspoint; import java.util.Enumeration; import java.util.HashSet; import java.util.ListResourceBundle; import java.util.Set; // create a class that extends to ListResourceBundle class MyResources extends ListResourceBundle { // get contents must be implemented protected Object[][] getContents() { return new Object[][] { {"1", "Hello World!"}, {"2", "Goodbye World!"}, {"3", "Goodnight World!"} }; } protected Set<String> handleKeySet() { Set<String> set = new HashSet<String>(); // get the keys Enumeration<String> enumeration = getKeys(); // print the keys while (enumeration.hasMoreElements()) { set.add(enumeration.nextElement()); } return set; } } public class ListResourceBundleDemo { public static void main(String[] args) { // create a new MyResources instance MyResources mr = new MyResources(); // get the set of Keys Set<String> keys = mr.handleKeySet(); // print each key keys.forEach(System.out::println); } }
输出
让我们编译并运行上述程序,这将产生以下结果。
1 2 3
获取字符串、对象对 ListResourceBundle 的键集示例
以下示例演示了 Java ListResourceBundle handleKeySet() 方法的使用,用于迭代 ListResourceBundle 的键。我们创建了一个 ListResourceBundle 对象,其内容为字符串、Student 对象对的二维数组。然后通过重写 getContents() 方法添加一些条目,然后在重写的 handleKeySet() 方法中检索键的枚举并进行迭代以获取键。在主方法中,我们使用 handleKeySet() 方法获取键的集合。
package com.tutorialspoint; import java.util.Enumeration; import java.util.HashSet; import java.util.ListResourceBundle; import java.util.Set; // create a class that extends to ListResourceBundle class MyResources extends ListResourceBundle { // get contents must be implemented protected Object[][] getContents() { return new Object[][] { {"1", new Student(1, "Julie")}, {"2", new Student(2, "Robert")}, {"3", new Student(3, "Adam")} }; } protected Set<String> handleKeySet() { Set<String> set = new HashSet<String>(); // get the keys Enumeration<String> enumeration = getKeys(); // print the keys while (enumeration.hasMoreElements()) { set.add(enumeration.nextElement()); } return set; } } public class ListResourceBundleDemo { public static void main(String[] args) { // create a new MyResources instance MyResources mr = new MyResources(); // get the set of Keys Set<String> keys = mr.handleKeySet(); // print each key keys.forEach(System.out::println); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
输出
让我们编译并运行上述程序,这将产生以下结果。
1 2 3