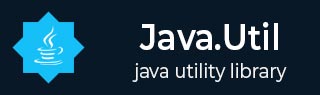
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Properties storeToXML(OutputStream os, String comment) 方法
描述
该 **Java Properties storeToXML(OutputStream os, String comment)** 方法会输出一个 XML 文档,该文档表示此表中包含的所有属性。 调用此方法的形式为 `props.storeToXML(os, comment)`,其行为与调用 `props.storeToXML(os, comment, "UTF-8")` 完全相同。
声明
以下是 **Java Properties storeToXML()** 方法的声明
public void storeToXML(OutputStream os,String comment)
参数
**out** − 用于输出 XML 文档的输出流。
**comments** − 属性列表的描述,如果不需要注释,则为 null。
返回值
此方法不返回值
异常
**IOException** − 如果将此属性列表写入指定的输出流引发 IOException。
**ClassCastException** − 如果此 Properties 对象包含任何非字符串的键或值。
**NullPointerException** − 如果 out 为 null。
Java Properties storeToXML(OutputStream os, String comment,String encoding) 方法
描述
该 **java.util.Properties.storeToXML(OutputStream os, String comment, String encoding)** 方法使用指定的编码输出一个 XML 文档,该文档表示此表中包含的所有属性。
声明
以下是 **java.util.Properties.storeToXML()** 方法的声明
public void storeToXML(OutputStream os,String comment, String encoding)
参数
**out** − 用于输出 XML 文档的输出流。
**comments** − 属性列表的描述,如果不需要注释,则为 null。
**encoding** − 受支持的字符编码的名称。
返回值
此方法不返回值
异常
**IOException** − 如果将此属性列表写入指定的输出流引发 IOException。
**ClassCastException** − 如果此 Properties 对象包含任何非字符串的键或值。
**NullPointerException** − 如果 os 或 encoding 为 null。
**UnsupportedEncodingException** − 如果实现不支持该编码。
Java Properties storeToXML(OutputStream os, String comment,Charset encoding) 方法
描述
该 **java.util.Properties.storeToXML(OutputStream os, String comment, Charset encoding)** 方法使用指定的编码输出一个 XML 文档,该文档表示此表中包含的所有属性。
声明
以下是 **java.util.Properties.storeToXML()** 方法的声明
public void storeToXML(OutputStream os,String comment, Charset encoding)
参数
**out** − 用于输出 XML 文档的输出流。
**comments** − 属性列表的描述,如果不需要注释,则为 null。
**charset** − 字符集。
返回值
此方法不返回值
异常
**IOException** − 如果将此属性列表写入指定的输出流引发 IOException。
**ClassCastException** − 如果此 Properties 对象包含任何非字符串的键或值。
**NullPointerException** − 如果 os 或 charset 为 null。
将 Properties 条目存储到 XML 文件的示例
以下示例演示了如何使用 Java Properties storeToXML(OutputStream os, String comment) 方法将属性对象存储到 xml 文件中,然后打印 xml。
package com.tutorialspoint; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.util.Properties; public class PropertiesDemo { public static void main(String[] args) { Properties prop = new Properties(); // add some properties prop.put("Height", "200"); prop.put("Width", "15"); try { // create a output and input as a xml file FileOutputStream fos = new FileOutputStream("properties.xml"); FileInputStream fis = new FileInputStream("properties.xml"); // store the properties in the specific xml prop.storeToXML(fos, "Properties Example"); // print the xml while (fis.available() > 0) { System.out.print("" + (char) fis.read()); } } catch (IOException ex) { ex.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE properties SYSTEM "http://java.sun.com/dtd/properties.dtd"> <properties> <comment>Properties Example</comment> <entry key="Height">200</entry> <entry key="Width">15</entry> </properties>
使用给定编码将 Properties 条目存储到 XML 文件的示例
以下示例演示了如何使用 Java Properties storeToXML(OutputStream os, String comment, String encoding) 方法使用给定编码将属性对象存储到 xml 文件中,然后打印 xml。
package com.tutorialspoint; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.util.Properties; public class PropertiesDemo { public static void main(String[] args) { Properties prop = new Properties(); // add some properties prop.put("Height", "200"); prop.put("Width", "15"); try { // create a output and input as a xml file FileOutputStream fos = new FileOutputStream("properties.xml"); FileInputStream fis = new FileInputStream("properties.xml"); // store the properties in the specific xml and a different encoding prop.storeToXML(fos, "Properties Example","windows-1252"); // print the xml. Notice that ISO 8859 isn't supported while (fis.available() > 0) { System.out.print("" + (char) fis.read()); } } catch (IOException ex) { ex.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
<?xml version="1.0" encoding="windows-1252"?> <!DOCTYPE properties SYSTEM "http://java.sun.com/dtd/properties.dtd"> <properties> <comment>Properties Example</comment> <entry key="Height">200</entry> <entry key="Width">15</entry> </properties>
使用默认编码将 Properties 条目存储到 XML 文件的示例
以下示例演示了如何使用 Java Properties storeToXML(OutputStream os, String comment, Charset encoding) 方法使用给定编码将属性对象存储到 xml 文件中,然后打印 xml。
package com.tutorialspoint; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.nio.charset.Charset; import java.util.Properties; public class PropertiesDemo { public static void main(String[] args) { Properties prop = new Properties(); // add some properties prop.put("Height", "200"); prop.put("Width", "15"); try { // create a output and input as a xml file FileOutputStream fos = new FileOutputStream("properties.xml"); FileInputStream fis = new FileInputStream("properties.xml"); // store the properties in the specific xml and a different encoding prop.storeToXML(fos, "Properties Example",Charset.defaultCharset()); // print the xml. while (fis.available() > 0) { System.out.print("" + (char) fis.read()); } } catch (IOException ex) { ex.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
<?xml version="1.0" encoding="windows-1252"?> <!DOCTYPE properties SYSTEM "http://java.sun.com/dtd/properties.dtd"> <properties> <comment>Properties Example</comment> <entry key="Height">200</entry> <entry key="Width">15</entry> </properties>