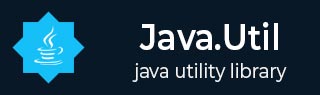
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包扩展
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Scanner findWithinHorizon() 方法
描述
Java Scanner findWithinHorizon(Pattern pattern,int horizon) 方法尝试查找指定模式的下一个出现位置。此方法搜索输入,直到指定的搜索范围,忽略分隔符。如果找到模式,则扫描程序将前进到匹配的输入并返回与模式匹配的字符串。如果未检测到此类模式,则返回 null,并且扫描程序的位置保持不变。此方法可能会阻塞,等待与模式匹配的输入。扫描程序永远不会在其当前位置之外搜索超过 horizon 代码点。请注意,匹配可能会被范围裁剪;也就是说,如果范围更大,则任意匹配结果可能会有所不同。
声明
以下是 java.util.Scanner.findWithinHorizon(Pattern pattern,int horizon) 方法的声明
public String findWithinHorizon(Pattern pattern,int horizon)
参数
pattern - 指定要搜索的模式的字符串
返回值
此方法返回与指定模式匹配的文本。
异常
IllegalStateException - 如果此扫描程序已关闭
IllegalArgumentException - 如果 horizon 为负数
Java Scanner findWithinHorizon(String pattern, int horizon) 方法
描述
Java Scanner findWithinHorizon(String pattern,int horizon) 方法尝试查找从指定字符串构建的模式的下一个出现位置,忽略分隔符。形式为 findWithinHorizon(pattern) 的此方法调用与调用 findWithinHorizon(Pattern.compile(pattern, horizon)) 的方式完全相同。
声明
以下是 java.util.Scanner.findWithinHorizon() 方法的声明
public String findWithinHorizon(String pattern,int horizon)
参数
pattern - 指定要搜索的模式的字符串
返回值
此方法返回与指定模式匹配的文本。
异常
IllegalStateException - 如果此扫描程序已关闭
IllegalArgumentException - 如果 horizon 为负数
使用 Scanner 在字符串上使用模式查找下一个标记的示例
以下示例演示了如何使用 Java Scanner findWithinHorizon(Pattern pattern, int horizon) 方法在给定字符串中查找模式的下一个出现位置。我们使用给定字符串创建了一个扫描程序对象。然后使用 findWithinHorizon(pattern, horizon) 在 10 的范围内搜索字符串,然后在 20 的范围内搜索模式。然后我们打印了字符串的其余部分。
package com.tutorialspoint; import java.util.Scanner; import java.util.regex.Pattern; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); // find a pattern of 2 letters before rld, with horizon of 10 System.out.println(scanner.findWithinHorizon(Pattern.compile("..rld"), 10)); // find a pattern of 2 letters before rld, with horizon of 20 System.out.println(scanner.findWithinHorizon(Pattern.compile("..rld"), 20)); // print the rest of the string System.out.println(scanner.nextLine()); // close the scanner scanner.close(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
null World ! 3 + 3.0 = 6
使用 Scanner 在字符串上使用字符串模式查找下一个标记的示例
以下示例演示了如何使用 Java Scanner findWithinHorizon(String pattern, int horizon) 方法在给定字符串中查找模式的下一个出现位置。我们使用给定字符串创建了一个扫描程序对象。然后使用 findWithinHorizon(pattern, horizon) 在 10 的范围内搜索字符串,然后在 20 的范围内搜索模式。然后我们打印了字符串的其余部分。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); // find a string of world, with horizon of 10 System.out.println(scanner.findWithinHorizon("World", 10)); // find a string of world, with horizon of 20 System.out.println(scanner.findWithinHorizon("World", 20)); // print the rest of the string System.out.println(scanner.nextLine()); // close the scanner scanner.close(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
null World ! 3 + 3.0 = 6
使用 Scanner 在用户输入上使用字符串模式查找下一个标记的示例
以下示例演示了如何使用 Java Scanner findWithinHorizon(String pattern, int horizon) 方法在给定输入中查找模式的下一个出现位置。我们使用 System.in 类创建了一个扫描程序对象。然后使用 findWithinHorizon(pattern, horizon) 在 10 的范围内搜索字符串,然后在 20 的范围内搜索模式。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with System input Scanner scanner = new Scanner(System.in); // find a string of world, with horizon of 10 System.out.println(scanner.findWithinHorizon("World", 10)); // find a string of world, with horizon of 20 System.out.println(scanner.findWithinHorizon("World", 20)); // close the scanner scanner.close(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:(我们在其中输入 Hello World 并按 Enter 键。)
Hello World null World