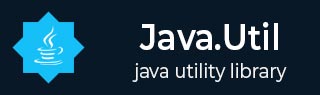
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Scanner hasNext() 方法
描述
java Scanner hasNext() 方法返回 true,如果此扫描器在其输入中还有另一个标记。此方法可能会阻塞,同时等待输入进行扫描。扫描器不会越过任何输入。
声明
以下是 java.util.Scanner.hasNext() 方法的声明
public boolean hasNext()
参数
无
返回值
当且仅当此扫描器有另一个标记时,此方法返回 true
异常
IllegalStateException − 如果此扫描器已关闭
Java Scanner hasNext(Pattern pattern) 方法
描述
java.util.Scanner.hasNext(Pattern pattern) 方法返回 true,如果下一个完整标记与指定的模式匹配。完整标记以匹配分隔符模式的输入为前缀和后缀。此方法可能会阻塞,同时等待输入。扫描器不会越过任何输入。
声明
以下是 java.util.Scanner.hasNext() 方法的声明
public boolean hasNext(Pattern pattern)
参数
pattern − 要扫描的模式
返回值
当且仅当此扫描器有另一个与指定模式匹配的标记时,此方法返回 true
异常
IllegalStateException − 如果此扫描器已关闭
Java Scanner hasNext(String pattern) 方法
描述
java.util.Scanner.hasNext(String pattern) 方法返回 true,如果下一个标记与从指定字符串构造的模式匹配。扫描器不会越过任何输入。形式为 hasNext(pattern) 的此方法调用与调用 hasNext(Pattern.compile(pattern)) 的行为完全相同。
声明
以下是 java.util.Scanner.hasNext() 方法的声明
public boolean hasNext(String pattern)
参数
pattern − 指定要扫描的模式的字符串
返回值
当且仅当此扫描器有另一个与指定模式匹配的标记时,此方法返回 true
异常
IllegalStateException − 如果此扫描器已关闭
使用字符串上的扫描器检查下一个标记是否可用示例
以下示例演示了 Java Scanner hasNext() 方法的使用,以检查下一个标记是否可用。我们使用给定的字符串创建了一个扫描器对象。然后,我们使用 hasNext() 方法检查了标记,然后我们使用 nextLine() 方法打印了字符串。现在,使用 hasNext() 检查是否还有更多标记,然后使用 close() 方法关闭扫描器。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); // check if the scanner has a token System.out.println("" + scanner.hasNext()); // print the rest of the string System.out.println("" + scanner.nextLine()); // check if the scanner has a token after printing the line System.out.println("" + scanner.hasNext()); // close the scanner scanner.close(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
true Hello World! 3 + 3.0 = 6 false
使用模式示例检查下一个标记是否可用
以下示例演示了 Java Scanner hasNext(Pattern pattern) 方法的使用,以检查下一个与给定模式匹配的标记是否可用。我们使用给定的字符串创建了一个扫描器对象。然后,我们使用 hasNext(pattern) 方法检查了标记,然后我们使用 nextLine() 方法打印了字符串。现在,使用 hasNext(pattern) 检查是否还有更多标记,然后使用 close() 方法关闭扫描器。
package com.tutorialspoint; import java.util.Scanner; import java.util.regex.Pattern; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); // check if the scanner's next token matches "rld" following 2 chars System.out.println("" + scanner.hasNext(Pattern.compile("..rld"))); // check if the scanner's next token matches "llo" following 2 chars System.out.println("" + scanner.hasNext(Pattern.compile("..llo"))); // print the rest of the string System.out.println("" + scanner.nextLine()); // close the scanner scanner.close(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
false true Hello World! 3 + 3.0 = 6
使用字符串作为模式的字符串上的扫描器示例检查下一个标记是否可用
以下示例演示了 Java Scanner hasNext(String pattern) 方法的使用,以检查下一个与给定模式匹配的标记是否可用。我们使用给定的字符串创建了一个扫描器对象。然后,我们使用 hasNext(pattern) 方法检查了标记,然后我们使用 nextLine() 方法打印了字符串。现在,使用 hasNext(pattern) 检查是否还有更多标记,然后使用 close() 方法关闭扫描器。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); // check if the scanner's next token matches "World" System.out.println("" + scanner.hasNext("World")); // check if the scanner's next token matches "Hello" System.out.println("" + scanner.hasNext("Hello")); // print the rest of the string System.out.println("" + scanner.nextLine()); // close the scanner scanner.close(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
false true Hello World! 3 + 3.0 = 6