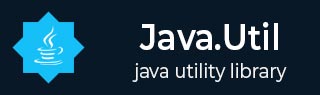
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包扩展
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Scanner hasNextByte() 方法
描述
Java Scanner hasNextByte() 方法返回 true,如果此扫描程序的输入中的下一个标记可以使用 nextByte() 方法解释为默认基数中的字节值。扫描程序不会前进到任何输入。
声明
以下是 java.util.Scanner.hasNextByte() 方法的声明
public boolean hasNextByte()
参数
无
返回值
当且仅当此扫描程序的下一个标记是有效的字节值时,此方法返回 true。
异常
IllegalStateException − 如果此扫描程序已关闭
使用 Scanner 在字符串上检查下一个标记是否为字节的示例
以下示例演示了 Java Scanner hasNextByte() 方法的使用,该方法使用默认基数检查下一个标记是否为字节。我们使用给定的字符串创建了一个扫描程序对象。然后我们检查每个标记是否是字节并打印。最后,使用 close() 方法关闭扫描程序。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); while (scanner.hasNext()) { // check if the scanner's next token is a Byte System.out.println("" + scanner.hasNextByte()); // print what is scanned System.out.println("" + scanner.next()); } // close the scanner scanner.close(); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
false Hello false World! true 3 false + false 3.0 false = true 6
使用 Scanner 在字符串上检查基数为 5 的下一个标记是否为字节的示例
以下示例演示了 Java Scanner hasNextByte() 方法的使用,该方法使用基数 5 检查下一个标记是否为字节。我们使用给定的字符串创建了一个扫描程序对象。然后我们检查每个标记是否是字节并打印。最后,使用 close() 方法关闭扫描程序。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); while (scanner.hasNext()) { // check if the scanner's next token is a Byte System.out.println("" + scanner.hasNextByte(4)); // print what is scanned System.out.println("" + scanner.next()); } // close the scanner scanner.close(); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
false Hello false World! true 3 false + false 3.0 false = false 6
使用 Scanner 在用户输入上检查下一个标记是否为字节的示例
以下示例演示了 Java Scanner hasNextByte() 方法的使用,该方法检查下一个标记是否为字节。我们使用 System.in 类创建了一个扫描程序对象。然后我们检查每个标记是否是字节并打印。最后,使用 close() 方法关闭扫描程序。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { // create a new scanner with System Input Scanner scanner = new Scanner(System.in); // check if the scanner's next token is a Byte if(scanner.hasNextByte()){ // print what is scanned System.out.println(scanner.next()); } else { scanner.next(); } // close the scanner scanner.close(); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:(我们在其中输入了 3.0。)
2 2
java_util_scanner.htm
广告