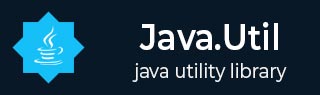
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包额外内容
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Scanner hasNextLine() 方法
描述
Java Scanner hasNextLine() 方法返回 true,如果此扫描器的输入中还有另一行。此方法在等待输入时可能会阻塞。扫描器不会越过任何输入。
声明
以下是 java.util.Scanner.hasNextLine() 方法的声明
public boolean hasNextLine()
参数
无
返回值
当且仅当此扫描器有另一行输入时,此方法返回 true
异常
IllegalStateException − 如果此扫描器已关闭
使用字符串示例检查扫描器中是否存在下一行
以下示例演示了 Java Scanner hasNextLine() 方法的使用,用于检查是否存在下一行。我们使用给定的字符串创建了一个扫描器对象。然后我们打印一行,然后使用 hasNextLine() 检查是否存在更多数据。一旦行结束,hasNextLine() 返回 false。最后,使用 close() 方法关闭扫描器。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! \n 3 + 3.0 = 6 "; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); // print the next line System.out.println(scanner.nextLine()); // check if there is a next line again System.out.println(scanner.hasNextLine()); // print the next line System.out.println(scanner.nextLine()); // check if there is a next line again System.out.println(scanner.hasNextLine()); // close the scanner scanner.close(); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
Hello World! true 3 + 3.0 = 6 false
使用用户输入示例检查扫描器中是否存在下一行
以下示例演示了 Java Scanner hasNextLine() 方法的使用,用于检查是否存在下一行。我们使用 System.in 创建了一个扫描器对象。然后我们打印一行,然后使用 hasNextLine() 检查是否存在更多数据。一旦行结束,hasNextLine() 返回 false。最后,使用 close() 方法关闭扫描器。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { // create a new scanner with the System Input Scanner scanner = new Scanner(System.in); // print the next line System.out.println(scanner.nextLine()); // check if there is a next line again System.out.println(scanner.hasNextLine()); // close the scanner scanner.close(); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:(我们在其中输入 Hello World 并按 Enter 键,然后输入 Bye 并按 Enter 键。)
Hello World Hello World Bye true
使用属性文件示例检查扫描器中是否存在下一行
以下示例演示了 Java Scanner hasNextLine() 方法的使用,用于检查是否存在下一行。我们使用文件 properties.txt 创建了一个扫描器对象。然后我们使用 hasNextLine() 方法检查每一行并打印。最后,使用 close() 方法关闭扫描器。
package com.tutorialspoint; import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) throws FileNotFoundException { // create a new scanner with a file as input Scanner scanner = new Scanner(new File("properties.txt")); // print the next line System.out.println(scanner.nextLine()); // check if there is a next line again System.out.println(scanner.hasNextLine()); // close the scanner scanner.close(); } }
假设我们在你的 CLASSPATH 中有一个名为 properties.txt 的文件,其内容如下。此文件将用作我们示例程序的输入:
Hello World! 3 + 3.0 = 6
输出
让我们编译并运行上面的程序,这将产生以下结果:
Hello World! 3 + 3.0 = 6 false