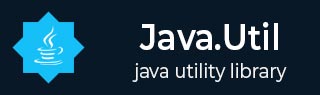
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包其他
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Scanner ioException() 方法
描述
java.Scanner ioException() 方法返回此 Scanner 的底层 Readable 最近抛出的 IOException。如果不存在此类异常,则此方法返回 null。
声明
以下是 java.util.Scanner.ioException() 方法的声明
public IOException ioException()
参数
无
返回值
此方法返回此扫描程序的可读对象最近抛出的异常
异常
无
使用字符串示例检查 Scanner 中的 ioException
以下示例演示了如何使用 Java Scanner ioException() 方法来检查此 Scanner 的底层 Readable 最近抛出的 IOException。我们使用给定的字符串创建了一个扫描程序对象。然后我们使用 nextLine() 方法打印字符串,然后打印任何异常。使用 close() 方法关闭 Scanner。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); // print the next line of the string System.out.println(scanner.nextLine()); System.out.println(scanner.ioException()); // close the scanner scanner.close(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Hello World! 3 + 3.0 = 6 null
使用用户输入示例检查 Scanner 中的 ioException
以下示例演示了如何使用 Java Scanner ioException() 方法来检查此 Scanner 的底层 Readable 最近抛出的 IOException。我们使用 System.in 类创建了一个扫描程序对象。然后我们使用 nextLine() 方法打印字符串,然后打印任何异常。然后使用 close() 方法关闭扫描程序。
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { // create a new scanner with the system input Scanner scanner = new Scanner(System.in); // print the next line of the string System.out.println(scanner.nextLine()); System.out.println(scanner.ioException()); // close the scanner scanner.close(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:(我们在其中输入 Hello World 并按 Enter 键。)
Hello World Hello World null
使用属性文件示例检查 Scanner 中的 ioException
以下示例演示了如何使用 Java Scanner ioException() 方法来检查此 Scanner 的底层 Readable 最近抛出的 IOException。我们使用文件 properties.txt 创建了一个扫描程序对象。然后我们使用 nextLine() 方法打印内容,然后打印任何异常。然后使用 close() 方法关闭扫描程序。
package com.tutorialspoint; import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) throws FileNotFoundException { // create a new scanner with a file as input Scanner scanner = new Scanner(new File("properties.txt")); // print the next line of the string System.out.println(scanner.nextLine()); System.out.println(scanner.ioException()); // close the scanner scanner.close(); } }
假设我们在你的 CLASSPATH 中有一个名为 properties.txt 的文件,其内容如下。此文件将用作我们示例程序的输入:
Height=200 Width=15
输出
让我们编译并运行上述程序,这将产生以下结果。
Height=200 null