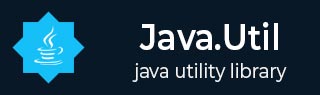
- Java.util 包类
- Java.util - 首页
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util 包扩展
- Java.util - 接口
- Java.util - 异常
- Java.util - 枚举
- Java.util 有用资源
- Java.util - 有用资源
- Java.util - 讨论
Java Timer cancel() 方法
描述
Java Timer cancel() 方法用于终止此计时器并丢弃任何当前计划的任务。
声明
以下是 java.util.Timer.cancel() 方法的声明。
public void cancel()
参数
无
返回值
无
异常
无
使用固定日期和初始延迟取消计时器示例
以下示例演示了 Java Timer cancel() 方法的使用,用于取消当前计划的计时器操作。我们使用 CustomTimerTask 对象创建了一个计时器对象。CustomTimerTask 是扩展 TimerTask 类并实现 run() 方法的自定义类,该方法将在计划时间执行。然后我们创建了一个计时器对象并使用 scheduleAtFixedRate() 方法计划了一个任务,该任务从现在开始每 100 毫秒执行一次。然后使用 cancel() 方法终止计时器操作。
package com.tutorialspoint; import java.util.Date; import java.util.Timer; import java.util.TimerTask; public class TimerDemo { public static void main(String[] args) { // creating timer task, timer TimerTask tasknew = new CustomTimerTask(); Timer timer = new Timer(); // scheduling the task timer.scheduleAtFixedRate(tasknew, new Date(), 100); // terminating the timer System.out.println("cancelling timer"); timer.cancel(); } } class CustomTimerTask extends TimerTask { @Override public void run() { System.out.println("working on"); } }
输出
让我们编译并运行以上程序,这将产生以下结果。
cancelling timer working on
将计时器计划为守护线程并取消示例
以下示例演示了 Java Timer cancel() 方法的使用,用于取消当前计划的计时器操作。我们使用 CustomTimerTask 对象创建了一个计时器对象。CustomTimerTask 是扩展 TimerTask 类并实现 run() 方法的自定义类,该方法将在计划时间执行。然后我们创建了一个作为守护线程的计时器对象,并使用 scheduleAtFixedRate() 方法计划了一个任务,该任务从现在开始每 100 毫秒执行一次。然后使用 cancel() 方法终止计时器操作。
package com.tutorialspoint; import java.util.Date; import java.util.Timer; import java.util.TimerTask; public class TimerDemo { public static void main(String[] args) { // creating timer task, timer TimerTask tasknew = new CustomTimerTask(); Timer timer = new Timer(true); // scheduling the task timer.scheduleAtFixedRate(tasknew, new Date(), 100); // terminating the timer System.out.println("cancelling timer"); timer.cancel(); } } class CustomTimerTask extends TimerTask { @Override public void run() { System.out.println("working on"); } }
输出
让我们编译并运行以上程序,这将产生以下结果。
cancelling timer working on
将命名计时器计划为守护线程并取消示例
以下示例演示了 Java Timer cancel() 方法的使用,用于取消当前计划的计时器操作。我们使用 CustomTimerTask 对象创建了一个计时器对象。CustomTimerTask 是扩展 TimerTask 类并实现 run() 方法的自定义类,该方法将在计划时间执行。然后我们创建了一个作为守护线程的计时器对象,并为其指定了一个名称,并使用 scheduleAtFixedRate() 方法计划了一个任务,该任务从现在开始每 100 毫秒执行一次。然后使用 cancel() 方法终止计时器操作。
package com.tutorialspoint; import java.util.Date; import java.util.Timer; import java.util.TimerTask; public class TimerDemo { public static void main(String[] args) { // creating timer task, timer TimerTask tasknew = new CustomTimerTask(); Timer timer = new Timer("test",true); // scheduling the task timer.scheduleAtFixedRate(tasknew, new Date(), 100); // terminating the timer System.out.println("cancelling timer"); timer.cancel(); } } class CustomTimerTask extends TimerTask { @Override public void run() { System.out.println("working on"); } }
输出
让我们编译并运行以上程序,这将产生以下结果。
cancelling timer working on